vue按需引入js文件
时间: 2023-07-01 10:06:54 浏览: 95
Vue.js的按需引入功能可以通过Webpack的Code Splitting特性实现,使用Webpack的import()函数动态加载组件或模块,从而实现按需引入。
举个例子,如果你想按需引入一个名为"my-component"的组件,可以在Vue的单文件组件中这样写:
```javascript
export default {
components: {
'my-component': () => import('./MyComponent.vue')
}
}
```
这样,在组件被渲染的时候才会动态加载对应的文件,从而实现按需引入。
当然,如果你使用的是Vue CLI,你可以使用它提供的@vue/cli-plugin-babel-plugin-component插件来自动化按需引入的过程。你只需要在Babel配置文件中添加如下代码:
```javascript
plugins: [
[
'component',
{
libraryName: 'your-library-name',
styleLibraryName: 'your-style-library-name'
}
]
]
```
然后在你需要引入组件的地方直接使用即可:
```javascript
import { MyComponent } from 'your-library-name'
Vue.use(MyComponent)
```
这样就可以自动按需引入组件了。
相关问题
vue如何引入js文件
在Vue中引入JS文件可以通过以下几种方式:
1. 在index.html文件中直接引入JS文件:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Vue App</title>
</head>
<body>
<div id="app"></div>
<script src="path/to/your/js/file.js"></script>
</body>
</html>
```
2. 使用Vue的插件机制,在main.js中引入JS文件并注册为插件:
```javascript
import yourPlugin from 'path/to/your/js/file.js'
Vue.use(yourPlugin)
```
3. 在Vue组件中使用import引入JS文件:
```javascript
import yourModule from 'path/to/your/js/file.js'
export default {
name: 'YourComponent',
methods: {
yourMethod() {
// 使用yourModule中的方法或变量
yourModule.someMethod()
const someVar = yourModule.someVar
}
}
}
```
vue怎么引入js文件
在Vue项目中引入JS文件,可以使用以下几种方式:
1. 在HTML文件中使用`<script>`标签引入JS文件,例如:
```html
<script src="./example.js"></script>
```
2. 在Vue组件中使用`import`语句引入JS文件,例如:
```javascript
import example from './example.js'
```
3. 在Vue的`index.html`文件中使用`<script>`标签引入JS文件,并在`main.js`中将其挂载到Vue实例上,例如:
```html
<script src="./example.js"></script>
```
```javascript
Vue.prototype.$example = example
```
无论使用哪种方式,都需要保证JS文件的路径正确,并且在Vue组件中使用引入的变量或函数时,需要在`methods`或`computed`中定义。
阅读全文
相关推荐
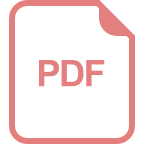
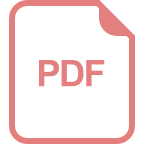
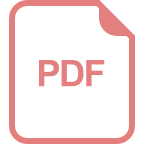








