使用Python实现DDRNet
时间: 2023-05-24 12:04:13 浏览: 88
由于DDRNet是基于PyTorch的深度学习模型,下面我们提供一个使用Python实现DDRNet的代码示例。
首先,我们需要安装 PyTorch 和 torchvision。如果您还没有安装,可以通过以下命令安装:
```
pip install torch torchvision
```
然后,我们可以通过以下代码加载DDRNet模型:
``` python
import torch
import torch.nn as nn
class DDRNet(nn.Module):
def __init__(self, num_classes=19):
super(DDRNet, self).__init__()
channels = [64, 128, 256, 512]
dilations = [[1, 1], [1, 1], [2, 2], [4, 4], [8, 8], [16, 16], [32, 32], [64, 64]]
self.conv1 = nn.Sequential(
nn.Conv2d(3, 32, kernel_size=3, stride=2, padding=1, bias=False),
nn.BatchNorm2d(32),
nn.ReLU(inplace=True),
nn.Conv2d(32, 32, kernel_size=3, stride=1, padding=1, bias=False),
nn.BatchNorm2d(32),
nn.ReLU(inplace=True),
nn.Conv2d(32, 64, kernel_size=3, stride=1, padding=1, bias=False),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
)
self.layer1 = nn.Sequential(
nn.Conv2d(64, channels[0], kernel_size=1, stride=1, padding=0, bias=False),
nn.BatchNorm2d(channels[0]),
nn.ReLU(inplace=True),
nn.Conv2d(channels[0], channels[0], kernel_size=3, stride=1, padding=dilations[0], dilation=dilations[0], bias=False),
nn.BatchNorm2d(channels[0]),
nn.ReLU(inplace=True),
)
self.layer2 = nn.ModuleList()
for i in range(2):
self.layer2.append(nn.Sequential(
nn.Conv2d(channels[0], channels[1], kernel_size=1, stride=1, padding=0, bias=False),
nn.BatchNorm2d(channels[1]),
nn.ReLU(inplace=True),
nn.Conv2d(channels[1], channels[1], kernel_size=3, stride=1, padding=dilations[i+1], dilation=dilations[i+1], bias=False),
nn.BatchNorm2d(channels[1]),
nn.ReLU(inplace=True),
))
self.layer3 = nn.ModuleList()
for i in range(3):
self.layer3.append(nn.Sequential(
nn.Conv2d(channels[1], channels[2], kernel_size=1, stride=1, padding=0, bias=False),
nn.BatchNorm2d(channels[2]),
nn.ReLU(inplace=True),
nn.Conv2d(channels[2], channels[2], kernel_size=3, stride=1, padding=dilations[i+3], dilation=dilations[i+3], bias=False),
nn.BatchNorm2d(channels[2]),
nn.ReLU(inplace=True),
))
self.layer4 = nn.ModuleList()
for i in range(2):
self.layer4.append(nn.Sequential(
nn.Conv2d(channels[2], channels[3], kernel_size=1, stride=1, padding=0, bias=False),
nn.BatchNorm2d(channels[3]),
nn.ReLU(inplace=True),
nn.Conv2d(channels[3], channels[3], kernel_size=3, stride=1, padding=dilations[i+6], dilation=dilations[i+6], bias=False),
nn.BatchNorm2d(channels[3]),
nn.ReLU(inplace=True),
))
self.context = nn.Sequential(
nn.Conv2d(channels[3], 512, kernel_size=3, stride=1, padding=1, bias=False),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
nn.Dropout2d(0.1),
nn.Conv2d(512, num_classes, kernel_size=1, stride=1, padding=0, bias=True),
)
def forward(self, x):
x = self.conv1(x)
x = self.layer1(x)
for layer in self.layer2:
x = x + layer(x)
for layer in self.layer3:
x = x + layer(x)
for layer in self.layer4:
x = x + layer(x)
x = self.context(x)
x = nn.functional.interpolate(x, scale_factor=8, mode='bilinear', align_corners=True)
return x
```
以上就完成了在 Python 中实现 DDRNet 的过程,我们可以通过以下方式加载预训练模型:
``` python
DDRNet_model = DDRNet()
DDRNet_model.load_state_dict(torch.load('DDRNet_23_slim.pth'))
DDRNet_model.eval()
```
加载预训练模型后,我们就可以使用 DDRNet 模型进行图像语义分割、图像分类等任务。
相关推荐
















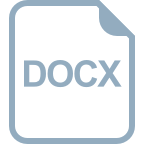