学生管理系统中通常都会涉及到学生类,请定义该类,包括学号、姓名、性别、年龄、班级、 Python 成绩、 JAVA 成绩、 Web 前端技术成绩等属性以及计算总成绩、平均成绩等方法;分别输出五位学生的完整信息,每名学生的总成绩、平均成绩, Python 成绩最高学生的姓名以及 Python 成绩的均值 +
时间: 2023-06-24 07:05:57 浏览: 85
以下是学生类的定义:
```python
class Student:
def __init__(self, id, name, gender, age, class_name, python_score, java_score, web_score):
self.id = id
self.name = name
self.gender = gender
self.age = age
self.class_name = class_name
self.python_score = python_score
self.java_score = java_score
self.web_score = web_score
def get_total_score(self):
return self.python_score + self.java_score + self.web_score
def get_average_score(self):
return self.get_total_score() / 3
```
接下来是五位学生的信息以及计算总成绩、平均成绩的代码:
```python
student1 = Student('1001', '张三', '男', 18, '一班', 90, 80, 85)
student2 = Student('1002', '李四', '女', 19, '一班', 85, 90, 80)
student3 = Student('1003', '王五', '男', 20, '二班', 95, 85, 90)
student4 = Student('1004', '赵六', '女', 18, '二班', 80, 95, 85)
student5 = Student('1005', '钱七', '男', 19, '三班', 90, 90, 90)
students = [student1, student2, student3, student4, student5]
for student in students:
print("学号:{}\n姓名:{}\n性别:{}\n年龄:{}\n班级:{}\nPython成绩:{}\nJAVA成绩:{}\nWeb前端技术成绩:{}\n".format(student.id, student.name, student.gender, student.age, student.class_name, student.python_score, student.java_score, student.web_score))
print("总成绩:{}\n平均成绩:{}\n".format(student.get_total_score(), student.get_average_score()))
```
最后,计算 Python 成绩最高学生的姓名以及 Python 成绩的均值:
```python
python_scores = [student.python_score for student in students]
max_python_score = max(python_scores)
max_python_student = next(student for student in students if student.python_score == max_python_score)
python_score_average = sum(python_scores) / len(python_scores)
print("Python成绩最高的学生是{},成绩为{}分".format(max_python_student.name, max_python_score))
print("Python成绩的平均值为{}分".format(python_score_average))
```
阅读全文
相关推荐
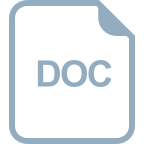
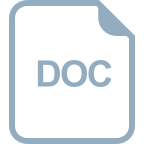
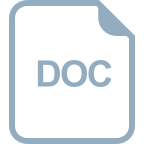



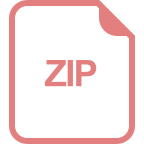
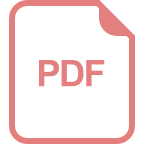
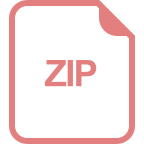
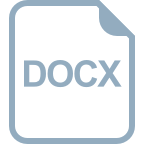
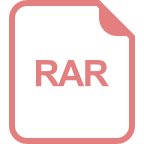
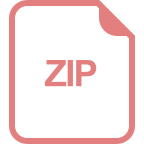
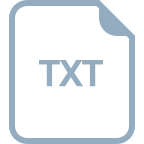
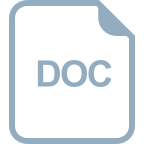
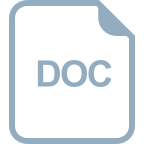
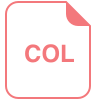



