C语言输入字符串判断英文字母的个数
时间: 2024-01-26 09:02:45 浏览: 29
可以使用C语言中的字符判断函数isalpha()来判断一个字符是否为英文字母,然后遍历输入的字符串,统计英文字母的个数即可。以下是一个示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main()
{
char str[100];
int i, count = 0;
printf("请输入一个字符串:\n");
scanf("%s", str);
for (i = 0; str[i] != '\0'; i++)
{
if (isalpha(str[i]))
{
count++;
}
}
printf("英文字母的个数为:%d\n", count);
return 0;
}
```
在这个示例代码中,我们使用了isalpha()函数来判断一个字符是否为英文字母,并使用一个循环遍历输入的字符串,对每个字符进行判断。如果该字符是英文字母,则计数器count加1。最后输出count的值即为英文字母的个数。
相关问题
代码生成c语言统计字符串中英文字母,空格,数字和其他字母的个数
以下是一个简单的代码示例,用于在C语言中统计字符串中的字母,空格,数字和其他字符的数量:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int i, alphabets, digits, spaces, others;
alphabets = digits = spaces = others = 0;
printf("Enter a string: ");
fgets(str, sizeof(str), stdin);
for(i=0; str[i]!='\0'; i++) {
if(isalpha(str[i])) {
alphabets++;
}
else if(isdigit(str[i])) {
digits++;
}
else if(isspace(str[i])) {
spaces++;
}
else {
others++;
}
}
printf("Total Alphabets = %d\n", alphabets);
printf("Total Digits = %d\n", digits);
printf("Total Spaces = %d\n", spaces);
printf("Total Other Characters = %d\n", others);
return 0;
}
```
运行示例:
```
Enter a string: Hello World! 123
Total Alphabets = 10
Total Digits = 3
Total Spaces = 2
Total Other Characters = 1
```
C语言编写函数统计一个字符串中英文字母的个数
可以使用C语言中的字符处理库函数`isalpha()`来判断一个字符是否是字母,然后遍历字符串,统计字母个数即可。下面是一个示例函数:
```c
#include <ctype.h>
int count_letters(char *str) {
int count = 0;
for (int i = 0; str[i] != '\0'; i++) {
if (isalpha(str[i])) {
count++;
}
}
return count;
}
```
函数`count_letters()`接受一个字符串指针作为参数,返回字符串中字母的个数。函数内部遍历字符串中的每个字符,如果是字母则计数器加1。最后返回计数器的值。注意需要在文件开头包含`ctype.h`头文件,以使用`isalpha()`函数。
相关推荐
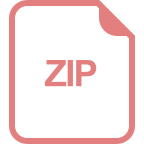
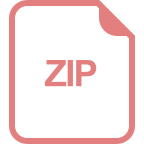
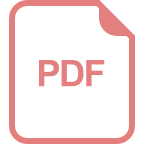
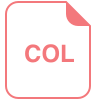
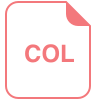
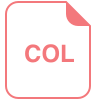
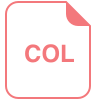
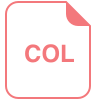







