請你幫我修改,我只需要指定文件夾當天生產的txt文檔進行匯總,不需要這個文件夾以前的也複製import os import shutil from datetime import date src_folder = r"Y:\iplas\Robot_Log\SMT" dst_folder = r"D:\測試log" today = date.today() # 創建目標文件夾(如果不存在) if not os.path.exists(dst_folder): os.makedirs(dst_folder) # 複製文件並重命名 for file_name in os.listdir(src_folder): if file_name.endswith('.txt'): file_path = os.path.join(src_folder, file_name) if date.fromtimestamp(os.path.getmtime(file_path)) == today: new_file_name = f"{today.strftime('%Y%m%d')}_{file_name}" shutil.copy(file_path, os.path.join(dst_folder, new_file_name)) # 寫入文件內容和相應文件名稱 file_contents = [] for file_name in os.listdir(dst_folder): if file_name.endswith('.txt'): try: with open(os.path.join(dst_folder, file_name), 'r', encoding='gbk') as t: file_content = t.read().strip() file_contents.append(f"{file_name[:-4]},{file_content}") except Exception as e: print(f"Error reading file {file_name}: {e}") # 將文件名稱改為"汇总文档.txt"並寫入文件 with open(os.path.join(dst_folder, '汇总文档.txt'), 'w', encoding='gbk') as f: f.write('\n'.join(file_contents))
时间: 2023-12-12 18:04:34 浏览: 101
以下是修改后的代码,只会处理当天生成的文本文件:
```python
import os
import shutil
from datetime import date
src_folder = r"Y:\iplas\Robot_Log\SMT"
dst_folder = r"D:\測試log"
today = date.today()
# 拼接当天的文件名
new_file_name = f"{today.strftime('%Y%m%d')}_汇总文档.txt"
new_file_path = os.path.join(dst_folder, new_file_name)
# 如果当天已经有汇总文档,则删除
if os.path.exists(new_file_path):
os.remove(new_file_path)
# 遍历源文件夹中的文件
for file_name in os.listdir(src_folder):
if file_name.endswith('.txt'):
file_path = os.path.join(src_folder, file_name)
# 如果文件是当天生成的,则复制到目标文件夹
if date.fromtimestamp(os.path.getmtime(file_path)) == today:
shutil.copy(file_path, dst_folder)
# 将源文件夹中当天生成的所有文件合并到汇总文档中
with open(new_file_path, 'w', encoding='gbk') as new_file:
for file_name in os.listdir(dst_folder):
if file_name.endswith('.txt'):
file_path = os.path.join(dst_folder, file_name)
# 如果文件是当天生成的,则将内容写入汇总文档中
if date.fromtimestamp(os.path.getmtime(file_path)) == today:
try:
with open(file_path, 'r', encoding='gbk') as t:
file_content = t.read().strip()
new_file.write(f"{file_name[:-4]},{file_content}\n")
except Exception as e:
print(f"Error reading file {file_name}: {e}")
```
修改的主要点:
1. 删除了创建目标文件夹的代码,因为如果目标文件夹不存在,`shutil.copy` 方法会自动创建它。
2. 在循环遍历源文件夹中的文件时,直接将当天生成的文件复制到目标文件夹中,不需要先重命名再复制。
3. 在将所有文件合并到汇总文档时,只处理当天生成的文件。同时,使用 `with open(new_file_path, 'w', encoding='gbk') as new_file:` 打开汇总文档,这样在写入完毕后会自动关闭文件,不需要手动调用 `new_file.close()`。另外,每次写入一行时,需要加上换行符 `\n`。
阅读全文
相关推荐
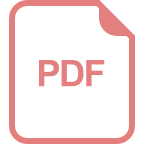
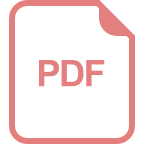
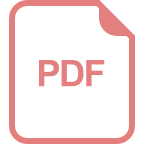
















