python的gui设计中var函数如何使用
时间: 2023-06-17 22:06:03 浏览: 98
在Python的GUI设计中,var函数通常用于创建与控制复选框、单选框等组件的变量。
具体来说,可以按照以下步骤使用var函数:
1. 导入所需的模块:
```
from tkinter import *
```
2. 创建一个Tkinter变量:
```
var = IntVar()
```
在上述代码中,IntVar()是一个Tkinter变量类,用于保存整数值。
3. 创建一个复选框或单选框,并将其关联到上述变量:
```
checkbox = Checkbutton(root, text='复选框', variable=var)
radiobutton = Radiobutton(root, text='单选框', variable=var)
```
在上述代码中,root是Tkinter窗口对象,text参数是复选框或单选框的文本标签,variable参数则是上述所创建的Tkinter变量。
4. 在需要时获取或修改变量的值:
```
value = var.get() # 获取变量值
var.set(1) # 设置变量值
```
在上述代码中,get()方法用于获取变量的值,set()方法用于设置变量的值。
需要注意的是,var函数的具体使用可能与不同的GUI库和版本有所不同,上述代码仅供参考。
相关问题
python gui 重命名文件
Python GUI重命名文件,一般是使用Tkinter模块来构建图形界面,同时利用os模块中的rename函数来完成文件重命名操作。
首先,需要在界面中添加一个文件选择按钮,在用户选中文件后,将文件路径保存到一个变量中。可以使用tkFileDialog模块来实现文件选择界面。
接下来再添加一个文本框,用于输入新的文件名。用户输入完毕后,保存到另一个变量中。
最后,添加一个“重命名”按钮,当用户点击该按钮时,将之前保存的文件路径和新文件名传递给rename函数,完成文件重命名操作。
需要注意的是,在重命名文件之前,最好先判断一下用户输入的新文件名是否已经存在,以避免文件名冲突。可以使用os模块中的exists函数判断文件是否存在。
示例代码如下:
```
import os
import tkinter as tk
from tkinter import filedialog
def select_file():
file_path = filedialog.askopenfilename()
file_path_var.set(file_path)
def rename_file():
file_path = file_path_var.get()
new_name = new_name_var.get()
if os.path.exists(new_name):
tk.messagebox.showerror("Error", "File name already exists!")
return
os.rename(file_path, new_name)
tk.messagebox.showinfo("Success", "File renamed successfully!")
root = tk.Tk()
root.title("Rename File")
root.geometry("400x200")
file_path_var = tk.StringVar()
new_name_var = tk.StringVar()
tk.Label(root, text="Select file to rename:").grid(row=0, column=0)
tk.Button(root, text="Select", command=select_file).grid(row=0, column=1)
tk.Label(root, textvariable=file_path_var).grid(row=1, column=0, columnspan=2)
tk.Label(root, text="Enter new file name:").grid(row=2, column=0)
tk.Entry(root, textvariable=new_name_var).grid(row=2, column=1)
tk.Button(root, text="Rename", command=rename_file).grid(row=3, column=0, columnspan=2)
root.mainloop()
```
使用python GUI实现任意文法消除左递归和回溯的算法程序
以下是使用Python Tkinter GUI实现任意文法消除左递归和回溯算法的示例代码:
```python
from tkinter import *
# Function to eliminate left recursion from grammar
def eliminate_left_recursion(grammar):
non_terminals = list(grammar.keys())
for i in range(len(non_terminals)):
for j in range(i):
A = non_terminals[i]
B = non_terminals[j]
productions = grammar[A]
new_productions = []
old_productions = []
for production in productions:
if production.startswith(B):
new_productions.append(production[1:] + A + "'")
else:
old_productions.append(production + A + "'")
if new_productions:
grammar[A] = old_productions
grammar[A + "'"] = new_productions
return grammar
# Function to eliminate left recursion and left factoring from grammar
def eliminate_left_recursion_and_left_factoring(grammar):
# Eliminate left recursion first
non_terminals = list(grammar.keys())
for i in range(len(non_terminals)):
for j in range(i):
A = non_terminals[i]
B = non_terminals[j]
productions = grammar[A]
new_productions = []
old_productions = []
for production in productions:
if production.startswith(B):
new_productions.append(production[1:] + A + "'")
else:
old_productions.append(production + A + "'")
if new_productions:
grammar[A] = old_productions
grammar[A + "'"] = new_productions
# Eliminate left factoring next
non_terminals = list(grammar.keys())
for A in non_terminals:
productions = grammar[A]
new_productions = []
old_productions = []
while len(productions) > 1:
alpha = set()
for production in productions:
alpha.add(production[0])
common_prefix = ''
for i in range(len(productions[0])):
if all(production[i] == productions[0][i] for production in productions):
common_prefix += productions[0][i]
else:
break
if common_prefix:
new_non_terminal = A + "'"
new_production = common_prefix + new_non_terminal
new_productions.append(new_production)
old_productions = [production[len(common_prefix):] for production in productions]
old_productions.append('')
grammar[A] = old_productions
grammar[new_non_terminal] = new_productions
productions = old_productions
else:
new_productions.append(productions.pop(0))
if productions:
new_productions.append(productions[0])
grammar[A] = new_productions
return grammar
# Function to display the grammar in the GUI
def display_grammar(grammar, text_widget):
text_widget.delete(1.0, END)
for non_terminal, productions in grammar.items():
text_widget.insert(END, non_terminal + " -> ")
for production in productions:
text_widget.insert(END, production + " | ")
text_widget.delete("end-2c", "end")
text_widget.insert(END, "\n")
# Function to handle button click event
def handle_click(grammar_textbox, result_textbox, eliminate_left_recursion_var, eliminate_left_factoring_var):
# Parse the grammar input
input_text = grammar_textbox.get(1.0, END).strip()
grammar = {}
for line in input_text.split('\n'):
non_terminal, productions = line.split(' -> ')
grammar[non_terminal] = [p.strip() for p in productions.split('|')]
# Eliminate left recursion and/or left factoring as requested
if eliminate_left_recursion_var.get():
grammar = eliminate_left_recursion(grammar)
if eliminate_left_factoring_var.get():
grammar = eliminate_left_recursion_and_left_factoring(grammar)
# Display the result
display_grammar(grammar, result_textbox)
# Create the GUI
root = Tk()
root.title("Eliminate Left Recursion and Left Factoring")
# Create the input box for the grammar
grammar_label = Label(root, text="Enter the grammar:")
grammar_label.pack()
grammar_textbox = Text(root, height=10)
grammar_textbox.pack()
# Create the checkboxes for left recursion and left factoring
eliminate_left_recursion_var = BooleanVar()
eliminate_left_recursion_checkbox = Checkbutton(root, text="Eliminate Left Recursion", variable=eliminate_left_recursion_var)
eliminate_left_recursion_checkbox.pack()
eliminate_left_factoring_var = BooleanVar()
eliminate_left_factoring_checkbox = Checkbutton(root, text="Eliminate Left Factoring", variable=eliminate_left_factoring_var)
eliminate_left_factoring_checkbox.pack()
# Create the button to perform the elimination
eliminate_button = Button(root, text="Eliminate", command=lambda: handle_click(grammar_textbox, result_textbox, eliminate_left_recursion_var, eliminate_left_factoring_var))
eliminate_button.pack()
# Create the output box for the result
result_label = Label(root, text="Result:")
result_label.pack()
result_textbox = Text(root, height=10)
result_textbox.pack()
# Start the GUI
root.mainloop()
```
该程序使用Tkinter库创建了一个简单的GUI窗口,其中包含一个文本框用于输入文法,两个复选框用于选择要执行的算法,一个按钮用于执行算法,并且另一个文本框用于显示结果。
算法本身实现为两个函数,一个用于仅消除左递归,另一个用于同时消除左递归和左公共因子。这些函数接受一个文法字典作为输入,并返回一个修改后的文法字典作为输出。
处理点击事件的函数从输入文本框中解析文法,根据复选框的状态选择要执行的算法,并将结果显示在输出文本框中。
最后,使用mainloop()函数启动GUI。
阅读全文
相关推荐
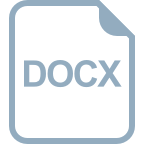
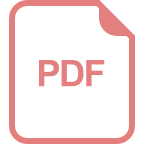
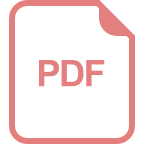
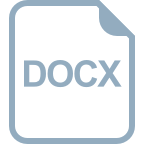
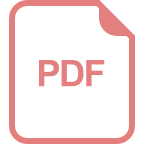
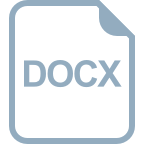
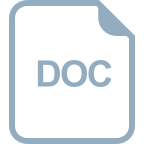
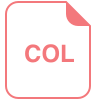
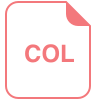
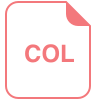
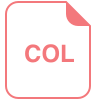
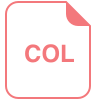
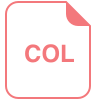
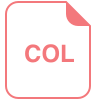
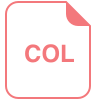