vue2.0如何实现将一行数据按相应的比例分成四份并且要设定最大值和最小值
时间: 2024-02-06 09:12:58 浏览: 64
可以使用flex布局来实现将一行数据按相应的比例分成四份,同时可以使用计算和watch来设定最大值和最小值。
1. 使用flex布局将一行数据按相应的比例分成四份:
在父元素上设置display: flex;,然后在子元素上设置相应的flex属性即可实现按比例分成四份。例如,要将一行数据分为2:1:1的比例,可以设置子元素的flex属性为2、1、1。
2. 设定最大值和最小值:
可以使用计算属性和watch来动态设定最大值和最小值。首先在data里面定义一个变量来存储最大值和最小值的范围,然后使用计算属性来计算实际的最大值和最小值,最后使用watch来监测变量的变化并更新数据。
具体实现可以参考下面的代码示例:
```html
<template>
<div class="container" :style="{ display: 'flex' }">
<div class="item" :style="{ flex: flex1 }">{{ data1 }}</div>
<div class="item" :style="{ flex: flex2 }">{{ data2 }}</div>
<div class="item" :style="{ flex: flex3 }">{{ data3 }}</div>
<div class="item" :style="{ flex: flex4 }">{{ data4 }}</div>
</div>
</template>
<script>
export default {
data() {
return {
data1: 10,
data2: 20,
data3: 30,
data4: 40,
range: [0, 100], // 最大值和最小值的范围
flex1: 2,
flex2: 1,
flex3: 1,
flex4: 1,
};
},
computed: {
// 计算实际的最大值和最小值
actualRange() {
const [min, max] = this.range;
const total = this.data1 + this.data2 + this.data3 + this.data4;
const maxRange = Math.min(max, total);
const minRange = Math.max(min, total - maxRange);
return [minRange, maxRange];
},
},
watch: {
// 监测变量的变化并更新数据
actualRange(newVal) {
const [min, max] = newVal;
const total = this.data1 + this.data2 + this.data3 + this.data4;
const ratio = total / (max - min);
this.flex1 = this.data1 / ratio;
this.flex2 = this.data2 / ratio;
this.flex3 = this.data3 / ratio;
this.flex4 = this.data4 / ratio;
},
},
};
</script>
<style>
.container {
width: 100%;
height: 100px;
}
.item {
height: 100%;
box-sizing: border-box;
border: 1px solid #ccc;
text-align: center;
}
</style>
```
阅读全文
相关推荐
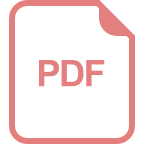
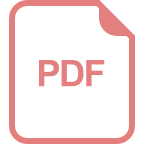
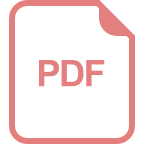
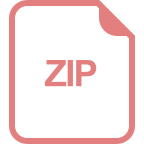
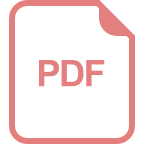
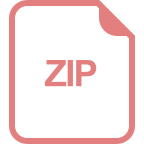
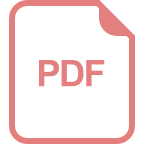
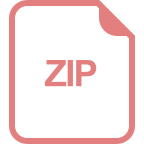
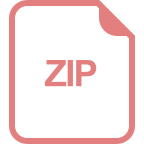
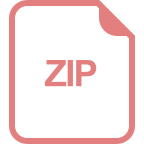
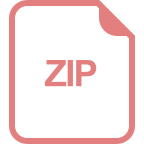
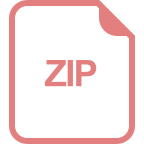
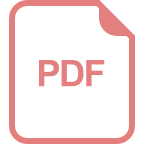
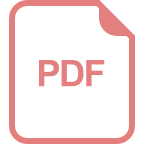
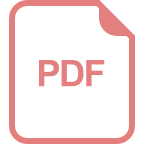
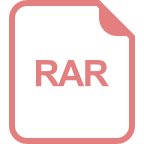
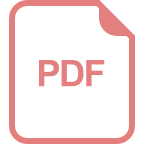