编写程序,建立2个带头结点单链表,输入若干整数将正整数插入第1个单链表,将负整数插入第2个单链表,插入前和插入后单链表保持递增或相等次序,显示2个单链表,最后销毁。
时间: 2024-03-23 13:17:44 浏览: 47
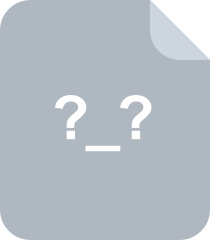
单链表的创建、插入、删除程序
好的,这是一个关于编程的问题。我可以为您提供代码实现,以下是 C++ 语言的代码示例:
```cpp
#include <iostream>
using namespace std;
struct Node {
int data;
Node* next;
Node(int d) : data(d), next(NULL) {}
};
class LinkedList {
private:
Node* head;
public:
LinkedList() : head(new Node(0)) {}
// 插入正整数节点到第1个单链表
void insertPositive(int x) {
Node* p = head;
while (p->next != NULL && p->next->data < x) {
p = p->next;
}
Node* q = new Node(x);
q->next = p->next;
p->next = q;
}
// 插入负整数节点到第2个单链表
void insertNegative(int x) {
Node* p = head->next;
Node* q = head;
while (p != NULL && p->data < x) {
q = p;
p = p->next;
}
Node* r = new Node(x);
r->next = p;
q->next = r;
}
// 显示2个单链表
void display() {
Node* p = head->next;
while (p != NULL) {
cout << p->data << " ";
p = p->next;
}
cout << endl;
}
// 销毁2个单链表
void destroy() {
Node* p = head->next;
while (p != NULL) {
Node* q = p;
p = p->next;
delete q;
}
head->next = NULL;
}
};
int main() {
LinkedList list1, list2;
int n, x;
cin >> n;
for (int i = 0; i < n; i++) {
cin >> x;
if (x > 0) {
list1.insertPositive(x);
} else {
list2.insertNegative(x);
}
}
list1.display();
list2.display();
list1.destroy();
list2.destroy();
return 0;
}
```
程序的思路是,首先创建两个带头结点的单链表,然后读入若干个整数,将正整数插入第1个单链表,将负整数插入第2个单链表,插入时保持单链表的递增顺序,最后分别输出两个单链表并销毁它们。
阅读全文
相关推荐
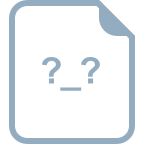








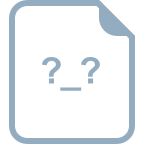
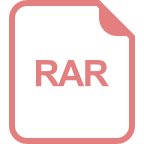
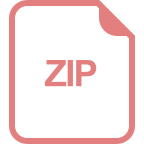
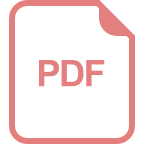
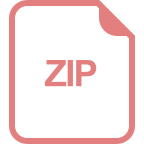
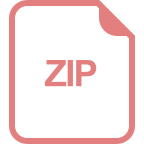