Files 和 Path SocketServer Socket 写一个 服务端发送文件1.jpg 和 客户端接收文件,并保存为2.jpg 用 java写
时间: 2024-05-01 14:20:57 浏览: 17
以下是一个简单的 Java 文件传输服务器和客户端示例,使用了 Files、Path、SocketServer 和 Socket 类:
服务端(Server)代码:
```java
import java.io.*;
import java.net.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class Server {
public static void main(String[] args) {
try {
ServerSocket serverSocket = new ServerSocket(1234);
System.out.println("Server is running and waiting for connection...");
Socket socket = serverSocket.accept();
System.out.println("Client connected.");
InputStream inputStream = socket.getInputStream();
// 读取文件名
byte[] fileNameBytes = new byte[1024];
int fileNameLength = inputStream.read(fileNameBytes);
String fileName = new String(fileNameBytes, 0, fileNameLength);
System.out.println("Received file name: " + fileName);
// 读取文件内容
byte[] fileContentBytes = new byte[1024];
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
int bytesRead;
while ((bytesRead = inputStream.read(fileContentBytes)) != -1) {
byteArrayOutputStream.write(fileContentBytes, 0, bytesRead);
}
byteArrayOutputStream.flush();
// 保存文件
Path outputFilePath = Paths.get("2.jpg");
Files.write(outputFilePath, byteArrayOutputStream.toByteArray());
System.out.println("File saved as: " + outputFilePath.toAbsolutePath().toString());
// 关闭连接
socket.close();
serverSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
客户端(Client)代码:
```java
import java.io.*;
import java.net.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class Client {
public static void main(String[] args) {
try {
Socket socket = new Socket("localhost", 1234);
System.out.println("Connected to server.");
OutputStream outputStream = socket.getOutputStream();
// 发送文件名
String fileName = "1.jpg";
byte[] fileNameBytes = fileName.getBytes();
outputStream.write(fileNameBytes);
outputStream.flush();
System.out.println("Sent file name: " + fileName);
// 发送文件内容
Path inputFilePath = Paths.get(fileName);
byte[] fileContentBytes = Files.readAllBytes(inputFilePath);
outputStream.write(fileContentBytes);
outputStream.flush();
System.out.println("Sent file content.");
// 关闭连接
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这个代码简单的示例只传输了一个图片文件,你可以根据需求修改代码来传输其他类型的文件或传输多个文件。
相关推荐
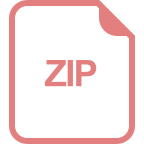
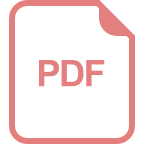














