用c++写一个学生类,包括编号和姓名,有添加和读取的操作,添加信息时编号不能重复,并排序后保存,读取
时间: 2024-03-01 20:53:05 浏览: 57
好的,这里是一个简单的C++代码,实现了您所需要的学生类的添加和读取操作:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
// 学生类
class Student {
private:
int id;
string name;
public:
Student(int id, string name) {
this->id = id;
this->name = name;
}
// 获取学生编号
int getId() const {
return this->id;
}
// 获取学生姓名
string getName() const {
return this->name;
}
// 重载 < 运算符,以便排序
bool operator < (const Student& other) const {
return this->id < other.id;
}
};
// 学生信息管理类
class StudentManager {
private:
vector<Student> students;
string filePath;
// 加载学生信息
void load() {
ifstream ifs(this->filePath);
while (!ifs.eof()) {
int id;
string name;
ifs >> id >> name;
if (id > 0 && name.size() > 0) {
Student student(id, name);
this->students.push_back(student);
}
}
ifs.close();
}
// 保存学生信息
void save() {
sort(this->students.begin(), this->students.end());
ofstream ofs(this->filePath);
for (auto student : this->students) {
ofs << student.getId() << " " << student.getName() << endl;
}
ofs.close();
}
public:
StudentManager(string filePath) {
this->filePath = filePath;
this->load();
}
~StudentManager() {
this->save();
}
// 添加学生信息
bool addStudent(Student student) {
for (auto s : this->students) {
if (s.getId() == student.getId()) {
return false;
}
}
this->students.push_back(student);
return true;
}
// 获取全部学生信息
vector<Student> getStudents() const {
return this->students;
}
};
int main() {
StudentManager manager("students.txt");
// 添加学生信息
manager.addStudent(Student(1001, "张三"));
manager.addStudent(Student(1002, "李四"));
manager.addStudent(Student(1003, "王五"));
manager.addStudent(Student(1002, "赵六")); // 添加重复编号的学生信息
// 获取全部学生信息并输出
vector<Student> students = manager.getStudents();
for (auto student : students) {
cout << student.getId() << " " << student.getName() << endl;
}
return 0;
}
```
注意,这里的学生信息是以文本文件的形式存储的,每一行包括一个学生的编号和姓名,中间用空格隔开。在程序运行时,会自动从文件中加载学生信息,并在程序结束时保存学生信息。
阅读全文
相关推荐

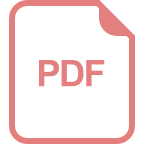
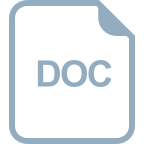
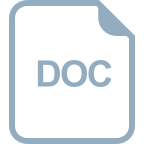
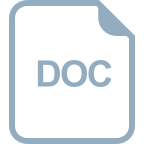
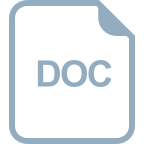
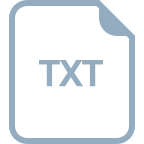
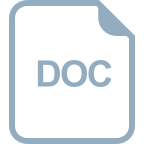
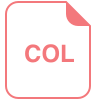
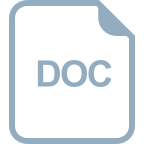
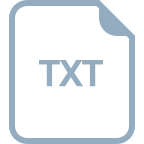
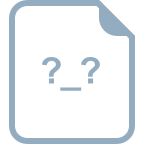
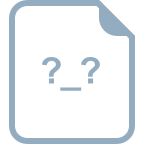
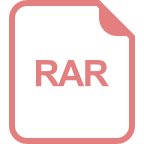
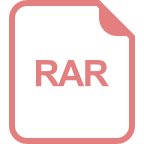