请给出C++使用OpenCV将视频每一帧图片中固定字幕以图片形式保存的代码
时间: 2024-05-09 17:14:20 浏览: 141
以下是使用OpenCV将视频每一帧图片中固定字幕以图片形式保存的C++代码:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
#include <string>
using namespace cv;
using namespace std;
int main()
{
// 打开视频文件
VideoCapture cap("video.mp4");
// 检查视频是否打开
if (!cap.isOpened())
{
cerr << "Error opening video file" << endl;
return -1;
}
// 获取视频的帧率、宽度和高度
double fps = cap.get(CAP_PROP_FPS);
int width = cap.get(CAP_PROP_FRAME_WIDTH);
int height = cap.get(CAP_PROP_FRAME_HEIGHT);
// 创建一个用于保存视频帧的Mat对象
Mat frame;
// 创建一个用于绘制字幕的Mat对象
Mat subtitle(height, width, CV_8UC3, Scalar(255, 255, 255));
// 设置字幕的文本和字体
string text = "Hello, world!";
int fontFace = FONT_HERSHEY_SIMPLEX;
double fontScale = 1;
int thickness = 2;
int baseline = 0;
Size textSize = getTextSize(text, fontFace, fontScale, thickness, &baseline);
// 计算字幕的位置
Point textOrg((width - textSize.width) / 2, (height + textSize.height) / 2);
// 循环读取视频帧
while (cap.read(frame))
{
// 将字幕绘制到视频帧上
rectangle(subtitle, textOrg + Point(0, baseline), textOrg + Point(textSize.width, -textSize.height), Scalar(0, 0, 0), FILLED);
putText(subtitle, text, textOrg, fontFace, fontScale, Scalar(255, 255, 255), thickness);
// 将字幕和视频帧叠加起来
Mat result;
addWeighted(frame, 1, subtitle, 0.5, 0, result);
// 保存叠加后的图像
string filename = "frame_" + to_string(cap.get(CAP_PROP_POS_FRAMES)) + ".jpg";
imwrite(filename, result);
}
// 释放视频流
cap.release();
return 0;
}
```
注意,以上代码假设你已经安装了OpenCV库,并且将视频文件命名为"video.mp4",可以根据需要进行修改。
阅读全文
相关推荐


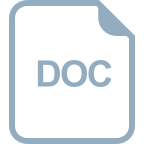

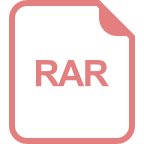
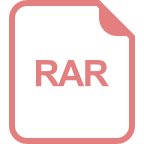
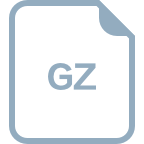
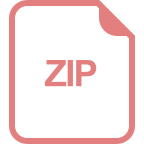
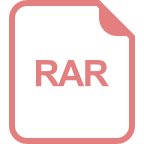
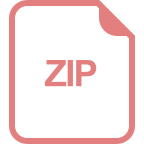
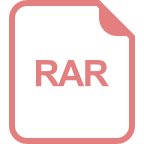
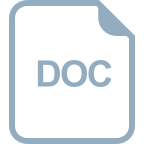
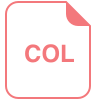
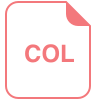
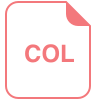