GD32F303 软件模拟I2C
时间: 2023-08-31 18:12:32 浏览: 113
以下是 GD32F303 软件模拟 I2C 的示例代码:
``` c
#include "gd32f30x.h"
#include "i2c_sw.h"
#define I2C_SCL_PIN GPIO_PIN_10
#define I2C_SDA_PIN GPIO_PIN_11
#define I2C_GPIO_PORT GPIOB
#define I2C_SCL_HIGH() GPIO_SetBits(I2C_GPIO_PORT, I2C_SCL_PIN)
#define I2C_SCL_LOW() GPIO_ResetBits(I2C_GPIO_PORT, I2C_SCL_PIN)
#define I2C_SDA_HIGH() GPIO_SetBits(I2C_GPIO_PORT, I2C_SDA_PIN)
#define I2C_SDA_LOW() GPIO_ResetBits(I2C_GPIO_PORT, I2C_SDA_PIN)
#define I2C_SDA_READ() GPIO_ReadInputDataBit(I2C_GPIO_PORT, I2C_SDA_PIN)
void i2c_sw_delay(void)
{
volatile uint32_t i;
for(i=0; i<100; i++);
}
void i2c_sw_init(void)
{
GPIO_InitPara GPIO_InitStructure;
RCC_AHBPeriphClock_Enable(RCC_AHBPERIPH_GPIOB);
GPIO_InitStructure.GPIO_Pin = I2C_SCL_PIN | I2C_SDA_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_MODE_OUT;
GPIO_InitStructure.GPIO_Speed = GPIO_SPEED_50MHZ;
GPIO_InitStructure.GPIO_OType = GPIO_OTYPE_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PUPD_PULLUP;
GPIO_Init(I2C_GPIO_PORT, &GPIO_InitStructure);
I2C_SCL_HIGH();
I2C_SDA_HIGH();
}
void i2c_sw_start(void)
{
I2C_SDA_HIGH();
I2C_SCL_HIGH();
i2c_sw_delay();
I2C_SDA_LOW();
i2c_sw_delay();
I2C_SCL_LOW();
}
void i2c_sw_stop(void)
{
I2C_SDA_LOW();
I2C_SCL_HIGH();
i2c_sw_delay();
I2C_SDA_HIGH();
i2c_sw_delay();
}
void i2c_sw_write_byte(uint8_t data)
{
uint8_t i;
for(i=0; i<8; i++)
{
if(data & 0x80)
I2C_SDA_HIGH();
else
I2C_SDA_LOW();
i2c_sw_delay();
I2C_SCL_HIGH();
i2c_sw_delay();
I2C_SCL_LOW();
i2c_sw_delay();
data <<= 1;
}
I2C_SDA_HIGH();
i2c_sw_delay();
I2C_SCL_HIGH();
i2c_sw_delay();
I2C_SCL_LOW();
}
uint8_t i2c_sw_read_byte(void)
{
uint8_t i, data = 0;
I2C_SDA_HIGH();
for(i=0; i<8; i++)
{
data <<= 1;
I2C_SCL_HIGH();
i2c_sw_delay();
if(I2C_SDA_READ() != RESET)
data |= 0x01;
I2C_SCL_LOW();
i2c_sw_delay();
}
return data;
}
void i2c_sw_write(uint8_t addr, uint8_t reg, uint8_t data)
{
i2c_sw_start();
i2c_sw_write_byte(addr << 1);
i2c_sw_write_byte(reg);
i2c_sw_write_byte(data);
i2c_sw_stop();
}
uint8_t i2c_sw_read(uint8_t addr, uint8_t reg)
{
uint8_t data;
i2c_sw_start();
i2c_sw_write_byte(addr << 1);
i2c_sw_write_byte(reg);
i2c_sw_start();
i2c_sw_write_byte((addr << 1) | 0x01);
data = i2c_sw_read_byte();
i2c_sw_stop();
return data;
}
```
使用方法:
1. 在主函数中调用 `i2c_sw_init()` 进行初始化。
2. 调用 `i2c_sw_write()` 写入数据,或调用 `i2c_sw_read()` 读取数据。
3. 注意在每次读写之前先调用 `i2c_sw_start()`,读写完成后调用 `i2c_sw_stop()`。
相关推荐
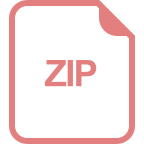

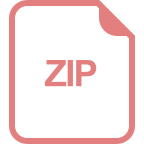
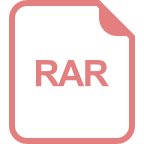
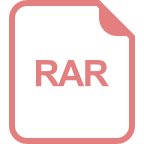




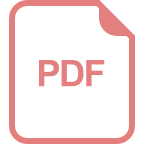
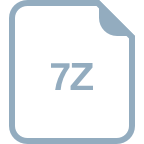
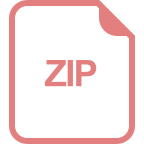
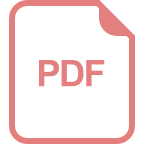
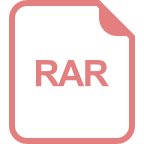
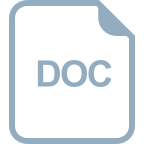