Django中怎么插入Python代码
时间: 2024-05-01 14:22:21 浏览: 205
在Django中插入Python代码有多种方式:
1. 在模板中使用模板标签:Django提供了一些内置的模板标签,如{% if %}、{% for %}等,可以在模板中直接使用。同时,也可以在自定义模板标签中插入Python代码。
2. 在视图函数中使用上下文变量:在视图函数中定义一个字典类型的上下文变量,然后将其传递给模板,在模板中可以使用该变量中的值。
3. 在模板中使用自定义过滤器:自定义过滤器是一种扩展Django模板引擎功能的方法,通过它可以对变量进行处理和格式化。在自定义过滤器中也可以插入Python代码。
例如,假设我们有一个视图函数,需要在模板中显示当前时间。可以在视图函数中定义一个字典类型的上下文变量:
```python
from datetime import datetime
def current_time(request):
now = datetime.now()
context = {'current_time': now}
return render(request, 'current_time.html', context)
```
然后在模板中使用该变量:
```html
<p>当前时间是:{{ current_time }}</p>
```
其中,`{{ current_time }}`会被替换成当前时间的字符串形式。
相关问题
Django 插入 Python 代码
在 Django 中插入 Python 代码的方式有很多种,以下是其中几种常用的方法:
1. 在模板中使用模板标签
Django 中提供了一系列的模板标签,可以在模板中执行 Python 代码。例如,使用 `{% if %}` 标签判断某个条件是否成立:
```html
{% if user.is_authenticated %}
<p>Welcome {{ user.username }}</p>
{% else %}
<p>Please log in</p>
{% endif %}
```
2. 在视图函数中执行 Python 代码
在视图函数中,可以执行任何 Python 代码,然后将结果传递给模板进行渲染。例如,在视图函数中获取当前时间,并将其传递给模板:
```python
from django.shortcuts import render
from datetime import datetime
def my_view(request):
now = datetime.now()
return render(request, 'my_template.html', {'now': now})
```
在模板中可以这样使用:
```html
<p>Current time is {{ now }}</p>
```
3. 在模型中定义方法
在 Django 中,模型可以定义方法,这些方法可以执行任何 Python 代码。例如,在模型中定义一个方法,返回当前用户的全部订单:
```python
from django.db import models
from django.contrib.auth.models import User
class Order(models.Model):
user = models.ForeignKey(User, on_delete=models.CASCADE)
product_name = models.CharField(max_length=100)
order_date = models.DateTimeField(auto_now_add=True)
def get_user_orders(self):
return Order.objects.filter(user=self.user)
```
在视图函数中可以这样使用:
```python
def my_view(request):
user = request.user
orders = user.order_set.all()
user_orders = []
for order in orders:
user_orders.extend(order.get_user_orders())
return render(request, 'my_template.html', {'user_orders': user_orders})
```
在模板中可以这样使用:
```html
{% for order in user_orders %}
<p>{{ order.product_name }} - {{ order.order_date }}</p>
{% endfor %}
```
以上是几种常用的在 Django 中插入 Python 代码的方法,具体使用哪种方法取决于具体的需求和场景。
Django怎么插入别的python代码
在 Django 中插入别的 Python 代码,可以使用以下几种方式:
1. 在 Django 视图函数中直接插入 Python 代码
视图函数中可以包含任何 Python 代码,这包括导入模块、定义函数、执行代码等。例如:
```python
from django.shortcuts import render
def my_view(request):
# 定义一个函数
def add(a, b):
return a + b
# 执行一些代码
x = 10
y = 20
result = add(x, y)
# 渲染模板并传递变量
return render(request, 'my_template.html', {'result': result})
```
2. 使用 Django 模板标签 `{% include %}` 插入 Python 代码
`{% include %}` 标签可以引入一个指定的模板文件,其中可以包含任何 Python 代码。例如:
```python
{% include 'my_python_code.py' %}
```
3. 在 Django 模型方法中插入 Python 代码
Django 模型方法可以包含任何 Python 代码,例如:
```python
from django.db import models
class MyModel(models.Model):
name = models.CharField(max_length=100)
age = models.PositiveIntegerField()
# 定义一个方法
def is_adult(self):
return self.age >= 18
```
在模板中可以使用 `{{ my_model.is_adult }}` 来调用该方法,并得到方法的返回值。
需要注意的是,在 Django 中插入 Python 代码要谨慎,尽量避免在模板中写过多的逻辑代码,以保证代码的可读性和可维护性。
阅读全文
相关推荐
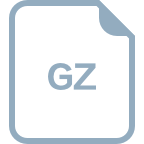


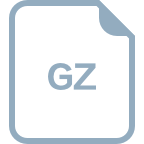








