用pytorch实现Our network has 3 layers of LSTM units followed by a final fully-connected layer of rectified linear units (ReLUs). A probability distribution for the next price move is produced by applying a softmax activation function.
时间: 2023-06-13 14:04:01 浏览: 45
可以使用PyTorch实现您所描述的神经网络,代码如下:
```python
import torch
import torch.nn as nn
class LSTMPredictor(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(LSTMPredictor, self).__init__()
self.hidden_size = hidden_size
self.lstm1 = nn.LSTM(input_size, hidden_size)
self.lstm2 = nn.LSTM(hidden_size, hidden_size)
self.lstm3 = nn.LSTM(hidden_size, hidden_size)
self.fc = nn.Linear(hidden_size, output_size)
self.softmax = nn.Softmax(dim=1)
def forward(self, input):
h1 = torch.zeros(1, input.size(1), self.hidden_size)
c1 = torch.zeros(1, input.size(1), self.hidden_size)
h2 = torch.zeros(1, input.size(1), self.hidden_size)
c2 = torch.zeros(1, input.size(1), self.hidden_size)
h3 = torch.zeros(1, input.size(1), self.hidden_size)
c3 = torch.zeros(1, input.size(1), self.hidden_size)
out1, (h1, c1) = self.lstm1(input, (h1, c1))
out2, (h2, c2) = self.lstm2(out1, (h2, c2))
out3, (h3, c3) = self.lstm3(out2, (h3, c3))
out4 = self.fc(out3[-1])
out5 = self.softmax(out4)
return out5
```
在这个模型中,我们使用了三个LSTM层,每个LSTM层的输出作为下一个LSTM层的输入。最后,我们使用一个全连接层和softmax激活函数来生成下一个价格移动的概率分布。
您可以根据您的数据集和需要调整输入大小(input_size)、隐藏层大小(hidden_size)和输出大小(output_size)。
相关推荐
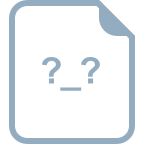
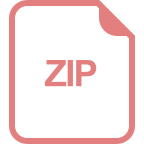
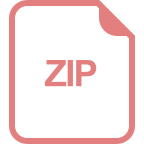
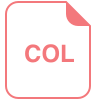













