当你输入很长的字符串时,会出现缓冲区溢出,在把name覆盖掉的同时,并且会更改gold的值为你的字符串后四位,请修改以下代码来实现:// savegame.c // Compile using gcc -fno-stack-protector savegame.c #include <stdio.h> #include <stdlib.h> #include <string.h> struct hero { unsigned int hp; char name[10]; unsigned int gold; }; void secret_function() { printf("You made it!\n"); return; } struct hero setup_hero() { struct hero h; h.hp = 30; h.gold = 8; scanf("%s", h.name); return h; } int main(int argc, const char *argv[]) { struct hero h = setup_hero(); printf("Name: %s\n", h.name); printf("HP: %d | Gold: %d\n", h.hp, h.gold); return 0; }
时间: 2023-06-27 12:02:12 浏览: 133
为了避免缓冲区溢出,可以使用 fgets() 函数代替 scanf() 函数读取输入。此外,可以使用 strncpy() 函数来复制字符串,以确保不会溢出。修改后的代码如下:
```
// savegame.c
// Compile using gcc -fno-stack-protector savegame.c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct hero {
unsigned int hp;
char name[10];
unsigned int gold;
};
void secret_function() {
printf("You made it!\n");
return;
}
struct hero setup_hero() {
struct hero h;
h.hp = 30;
h.gold = 8;
fgets(h.name, 10, stdin);
h.name[strcspn(h.name, "\n")] = '\0'; // Remove newline character from input
strncpy(&h.gold, &h.name[strlen(h.name) - 4], 4); // Copy last 4 characters of input into gold
return h;
}
int main(int argc, const char *argv[]) {
struct hero h = setup_hero();
printf("Name: %s\n", h.name);
printf("HP: %d | Gold: %d\n", h.hp, h.gold);
return 0;
}
```
在新的代码中,我们使用 fgets() 函数读取输入,并且使用 strcspn() 函数来删除输入中的换行符。然后,我们使用 strncpy() 函数将输入的最后四个字符复制到 gold 字段中。这样,即使输入的字符串很长,也不会溢出缓冲区。
相关推荐
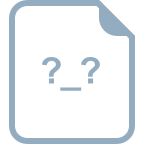
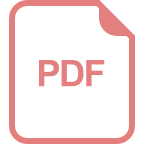
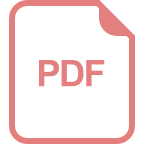














