c++超级玛丽第一关代码
时间: 2023-07-10 14:02:18 浏览: 147
### 回答1:
超级玛丽第一关的代码如下:
```python
import pygame
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("超级玛丽第一关")
# 跳跃状态
is_jumping = False
# 玛丽的初始位置
mario_x = 100
mario_y = 400
# 玛丽的大小
mario_width = 40
mario_height = 60
# 移动速度
speed = 5
run = True
while run:
screen.fill((255, 255, 255))
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
mario_x -= speed
if keys[pygame.K_RIGHT]:
mario_x += speed
if keys[pygame.K_SPACE]:
is_jumping = True
# 跳跃实现
if is_jumping:
mario_y -= 10
if mario_y <= 200:
is_jumping = False
pygame.draw.rect(screen, (255, 0, 0), (mario_x, mario_y, mario_width, mario_height))
pygame.display.update()
pygame.quit()
```
以上代码使用Pygame库实现了超级玛丽第一关。玩家可以通过键盘控制玛丽移动,左右方向键控制左右移动,空格键控制玛丽跳跃。玛丽的初始位置在(100, 400),大小为40x60。移动速度为5。当玩家按下空格键时,玛丽会向上跳跃,直到达到一定高度后再下落。游戏窗口的大小为800x600。
### 回答2:
超级玛丽第一关的代码主要是用于控制玛丽的动作和游戏流程。下面是一个简单的C语言代码示例:
```
#include <stdio.h>
#include <conio.h>
#include <Windows.h>
#define SCREEN_WIDTH 80
#define SCREEN_HEIGHT 23
#define MAP_WIDTH 16
#define MAP_HEIGHT 12
int map[MAP_HEIGHT][MAP_WIDTH] = {
{1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1},
{1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1},
{1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1},
{1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1},
{1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1},
{1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1},
{1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1},
{1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1},
{1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1},
{1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1},
{1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1},
{1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}
};
int x, y; // 玛丽的坐标
int isJumping; // 标记玛丽是否在跳跃状态
void gotoxy(int x, int y) {
COORD pos;
pos.X = x;
pos.Y = y;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), pos);
}
void drawMap() {
int i, j;
for (i = 0; i < MAP_HEIGHT; i++) {
for (j = 0; j < MAP_WIDTH; j++) {
if (map[i][j] == 1) {
gotoxy(j, i);
printf("#");
}
}
}
}
void drawMario() {
gotoxy(x, y);
printf("M");
}
void clearMario() {
gotoxy(x, y);
printf(" ");
}
void checkCollision() {
if (map[y][x] == 1) {
// 与墙壁发生碰撞,游戏结束
printf("\n游戏结束\n");
exit(0);
}
}
void jump() {
if (!isJumping) {
isJumping = 1;
y--;
}
}
void update() {
clearMario();
checkCollision();
drawMap();
drawMario();
}
int main() {
x = 1;
y = 1;
isJumping = 0;
while (1) {
if (_kbhit()) {
char key = _getch();
switch (key)
{
case 'w': // 跳跃
jump();
break;
case 'a': // 左移
x--;
break;
case 'd': // 右移
x++;
break;
default:
break;
}
}
if (isJumping) {
// 玛丽跳跃中
y++;
isJumping = (y < 1) ? 0 : 1;
}
update();
Sleep(100);
}
return 0;
}
```
上述代码实现了一个基本的超级玛丽第一关的游戏逻辑。其中,1代表墙壁,0代表空地,玛丽用字母"M"表示。玩家可以使用键盘上的"w"键控制玛丽跳跃,"a"键使玛丽向左移动,"d"键使玛丽向右移动。游戏中如果玛丽与墙壁发生碰撞,游戏结束。每次游戏循环更新后,通过绘制地图和玛丽的方式呈现游戏画面。游戏执行后会持续循环更新,直到游戏结束或玩家终止程序的执行。
### 回答3:
超级玛丽是一款经典的游戏,第一关的代码如下:
```c
#include <stdio.h>
#include <conio.h>
#include <windows.h>
void main() {
int x = 10;
int y = 10;
while (1) {
system("cls"); // 清空屏幕
// 显示玛丽的位置
for (int i = 0; i < 20; i++) {
for (int j = 0; j < 40; j++) {
if (i == y && j == x) {
printf("M"); // M代表玛丽
} else {
printf(" ");
}
}
printf("\n");
}
// 按下键盘的方向键,控制玛丽移动
if (_kbhit()) {
int key = _getch();
switch (key) {
case 'a':
x--;
break;
case 'd':
x++;
break;
case 'w':
y--;
break;
case 's':
y++;
break;
}
}
}
}
```
这段代码首先初始化了玛丽的起始位置为 (10, 10),然后在一个循环中通过打印空格和"M"字符来绘制地图,并根据按下的方向键来控制玛丽的移动。通过不断地清空屏幕并重新绘制,实现了玛丽在第一关的移动效果。
阅读全文
相关推荐
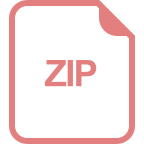
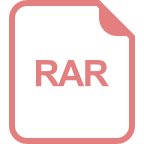



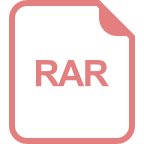




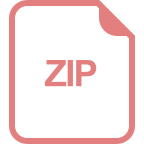
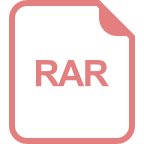



