一个电器销售公司想开发一套管理系统,该公司的主要业务是销售一些家用电器,例如:电冰箱、洗衣机、电视机产品。经调查,各种电器的属性如下: 冰箱类:品牌、型号、颜色、售价、门款式、制冷方式。 洗衣机类:品牌、型号、颜色、售价、电机类型、洗涤容量。电视类:品牌、型号、颜色、售价、屏幕尺寸、分辨率。不同的电器有不同的显示信息的方式。 要求:用封装、继承(包括this、super等)等知识点编程实现。
时间: 2023-07-03 21:10:43 浏览: 43
好的,这是一个比较典型的面向对象编程的场景,可以通过封装、继承等知识点来完成。以下是一个简单的示例代码:
```
// 家用电器类
class HomeAppliance {
protected String brand; // 品牌
protected String model; // 型号
protected String color; // 颜色
protected double price; // 售价
public HomeAppliance(String brand, String model, String color, double price) {
this.brand = brand;
this.model = model;
this.color = color;
this.price = price;
}
// 显示信息
public void showInfo() {
System.out.println("品牌:" + brand);
System.out.println("型号:" + model);
System.out.println("颜色:" + color);
System.out.println("售价:" + price);
}
}
// 冰箱类
class Refrigerator extends HomeAppliance {
private String doorStyle; // 门款式
private String coolingMethod; // 制冷方式
public Refrigerator(String brand, String model, String color, double price, String doorStyle, String coolingMethod) {
super(brand, model, color, price);
this.doorStyle = doorStyle;
this.coolingMethod = coolingMethod;
}
// 显示信息
@Override
public void showInfo() {
super.showInfo();
System.out.println("门款式:" + doorStyle);
System.out.println("制冷方式:" + coolingMethod);
}
}
// 洗衣机类
class WashingMachine extends HomeAppliance {
private String motorType; // 电机类型
private double washingCapacity; // 洗涤容量
public WashingMachine(String brand, String model, String color, double price, String motorType, double washingCapacity) {
super(brand, model, color, price);
this.motorType = motorType;
this.washingCapacity = washingCapacity;
}
// 显示信息
@Override
public void showInfo() {
super.showInfo();
System.out.println("电机类型:" + motorType);
System.out.println("洗涤容量:" + washingCapacity + "kg");
}
}
// 电视机类
class Television extends HomeAppliance {
private int screenSize; // 屏幕尺寸
private String resolution; // 分辨率
public Television(String brand, String model, String color, double price, int screenSize, String resolution) {
super(brand, model, color, price);
this.screenSize = screenSize;
this.resolution = resolution;
}
// 显示信息
@Override
public void showInfo() {
super.showInfo();
System.out.println("屏幕尺寸:" + screenSize + "英寸");
System.out.println("分辨率:" + resolution);
}
}
// 测试类
public class HomeApplianceTest {
public static void main(String[] args) {
// 创建一个冰箱对象
Refrigerator refrigerator = new Refrigerator("Haier", "BCD-123", "白色", 2999.0, "对开门", "直冷");
// 显示冰箱信息
System.out.println("冰箱信息:");
refrigerator.showInfo();
// 创建一个洗衣机对象
WashingMachine washingMachine = new WashingMachine("LG", "WM-456", "灰色", 3999.0, "变频电机", 8.0);
// 显示洗衣机信息
System.out.println("洗衣机信息:");
washingMachine.showInfo();
// 创建一个电视机对象
Television television = new Television("Sony", "KD-789", "黑色", 6999.0, 55, "4K");
// 显示电视机信息
System.out.println("电视机信息:");
television.showInfo();
}
}
```
在这个示例中,我们定义了一个`HomeAppliance`类作为所有家用电器的父类,其中包含了品牌、型号、颜色和售价等属性,并提供了一个`showInfo()`方法来显示信息。
然后,我们定义了三个子类`Refrigerator`、`WashingMachine`和`Television`,分别继承自`HomeAppliance`类,并添加了各自特有的属性和方法,同时重写了`showInfo()`方法来显示各自的信息。
最后,在测试类中,我们分别创建了一个冰箱对象、一个洗衣机对象和一个电视机对象,并分别调用它们的`showInfo()`方法来显示信息。
这样,我们就完成了一个简单的家用电器管理系统。
相关推荐
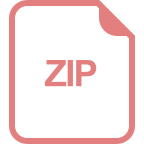
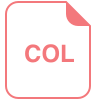
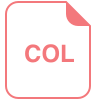
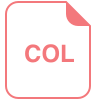
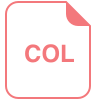










