python求最高值
时间: 2023-09-17 21:06:31 浏览: 39
### 回答1:
你可以使用Python内置的`max()`函数来找到一个列表或元组中的最大值,例如:
```python
my_list = [5, 3, 8, 1, 9]
max_value = max(my_list)
print(max_value) # 输出 9
```
如果你想找到多个参数中的最大值,也可以直接传入多个参数给`max()`函数:
```python
max_value = max(5, 3, 8, 1, 9)
print(max_value) # 输出 9
```
### 回答2:
要用Python求一组数中的最高值,可以使用以下方法:
1. 遍历列表:将要查找最高值的一组数存储在一个列表中,使用for循环遍历列表中的每个元素,找到最大值。
```python
numbers = [1, 5, 3, 9, 2]
max_value = numbers[0] # 假设列表中第一个元素为最大值
for num in numbers:
if num > max_value:
max_value = num
print("最大值为:", max_value)
```
2. 使用内置函数:Python提供了内置函数`max()`用于找到一组数中的最大值,可以直接使用该函数进行求解。
```python
numbers = [1, 5, 3, 9, 2]
max_value = max(numbers)
print("最大值为:", max_value)
```
这两种方法都可以用来求取一组数中的最大值,根据具体情况选择合适的方法即可。
### 回答3:
要使用Python求解一组数中的最高值,可以使用内置函数`max()`来实现。`max()`函数接受一个可迭代对象作为参数,返回其中的最大值。
假设有一个列表`numbers = [1, 5, 2, 9, 4]`,我们可以使用以下代码求解最高值:
```python
numbers = [1, 5, 2, 9, 4]
highest_value = max(numbers)
print("最高值为:" + str(highest_value))
```
输出结果为:
```
最高值为:9
```
在这个例子中,`max()`函数找到了列表`numbers`中的最高值9,然后将其赋值给变量`highest_value`。
除了列表,`max()`函数还可以用于元组、集合等其他可迭代对象。如果想求解多个参数中的最高值,也可以将这些参数传递给`max()`函数,例如:
```python
highest_value = max(1, 5, 2, 9, 4)
```
这样会得到相同的结果。
总之,使用Python求解一组数中的最高值可以使用内置函数`max()`,它简单方便且功能强大。
相关推荐
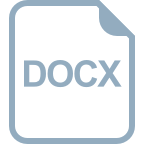














