写一段用python爬虫网易云音乐歌手粉丝数的代码
时间: 2023-07-14 11:11:51 浏览: 51
下面是一个简单的用Python爬虫网易云音乐歌手粉丝数的代码,需要安装requests和beautifulsoup4两个库:
```python
import requests
from bs4 import BeautifulSoup
# 歌手ID
artist_id = '6452'
# 构造请求头
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 发送HTTP请求
url = 'https://music.163.com/artist?id=' + artist_id
response = requests.get(url, headers=headers)
# 解析HTML
soup = BeautifulSoup(response.text, 'html.parser')
fans = soup.find('strong', {'id': 'fan_count'}).text
print('该歌手粉丝数为:' + fans)
```
将`artist_id`变量替换为你想要查询的歌手的ID即可。
相关问题
写一段python爬取网易云音乐歌手粉丝数的代码
好的,以下是爬取网易云音乐歌手粉丝数的Python代码:
```python
import requests
from bs4 import BeautifulSoup
# 输入歌手ID
artist_id = input("请输入歌手ID:")
# 构造URL
url = "https://music.163.com/artist?id=" + artist_id
# 发送GET请求
response = requests.get(url)
# 使用BeautifulSoup解析HTML
soup = BeautifulSoup(response.text, 'html.parser')
# 定位到粉丝数所在的标签
fans_tag = soup.find('strong', {'class': 'nb'})
# 输出粉丝数
print("粉丝数:", fans_tag.text)
```
使用方法:
1. 运行程序;
2. 输入歌手ID;
3. 程序会输出该歌手在网易云音乐的粉丝数。
注意:需要先安装requests和beautifulsoup4库。可以使用以下命令安装:
```bash
pip install requests beautifulsoup4
```
用Python写一段QQ音乐歌手信息爬虫代码
好的,以下是用Python写的QQ音乐歌手信息爬虫代码:
```python
import requests
from bs4 import BeautifulSoup
# 要爬取的歌手的名字
singer_name = "周杰伦"
# 构建请求头部
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"}
# 构建请求URL
url = "https://c.y.qq.com/soso/fcgi-bin/client_search_cp?&cr=1&p=1&n=20&w=" + singer_name
# 发送HTTP请求
response = requests.get(url, headers=headers)
# 解析响应数据
soup = BeautifulSoup(response.text, 'html.parser')
# 获取歌手信息列表
singer_list = soup.find_all('li', class_='singer_list')
# 打印歌手信息
for singer in singer_list:
singer_name = singer.find('h3').get_text()
singer_info = singer.find('p').get_text()
print(singer_name + " - " + singer_info)
```
接下来,请问您还有其他问题吗?
相关推荐
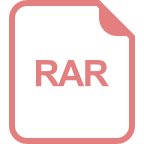
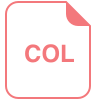
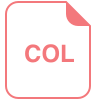
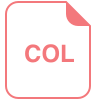
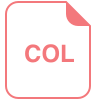
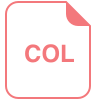







