python代码写一个图像查看器
时间: 2023-08-31 21:22:14 浏览: 202
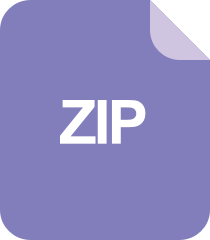
AscentViewer:用Python编写的图像查看器
### 回答1:
好的,这是一个使用 Python 写的图像查看器的示例代码:
```
import os
from tkinter import Tk, Label, Button
from PIL import Image, ImageTk
class ImageViewer:
def __init__(self, root, file_path):
self.root = root
self.file_path = file_path
# 初始化窗口
self.root.title("图像查看器")
self.root.geometry("600x400")
self.root.resizable(False, False)
# 初始化控件
self.label = Label(self.root)
self.label.pack()
self.prev_button = Button(self.root, text="上一张", command=self.prev_image)
self.prev_button.pack()
self.next_button = Button(self.root, text="下一张", command=self.next_image)
self.next_button.pack()
# 加载图像
self.images = []
for file in os.listdir(self.file_path):
if file.endswith(".jpg") or file.endswith(".png"):
image = Image.open(os.path.join(self.file_path, file))
self.images.append(image)
self.total_images = len(self.images)
self.current_image = 0
self.display_image()
def prev_image(self):
self.current_image -= 1
if self.current_image < 0:
self.current_image = self.total_images - 1
self.display_image()
def next_image(self):
self.current_image += 1
if self.current_image >= self.total_images:
self.current_image = 0
self.display_image()
def display_image(self):
image = self.images[self.current_image]
image = ImageTk.PhotoImage(image)
self.label.config(image=image)
self.label.image = image
if __name__ == "__main__":
root = Tk()
viewer = ImageViewer(root, "images")
root.mainloop()
```
这个图像查看器的功能是:通过点击按钮浏览图像文件夹中的图像,并在窗口中显示当前图像。
这段代码使用了 Tkinter 库来创建图形界面,使用 PIL 库来打开
### 回答2:
图像查看器是一种用于展示图片的软件程序。Python提供了许多库和模块来处理图像,其中一个常用的库是PIL(Python Imaging Library)。
首先,我们需要安装PIL库。可以使用pip命令在终端中输入以下指令进行安装:
```
pip install pillow
```
安装完PIL库后,我们可以开始编写代码。以下是一个简单的图像查看器的Python代码示例:
```python
from PIL import Image
# 选择要查看的图像文件
image_file = "image.jpg" # 图像文件的路径
# 打开图像文件
image = Image.open(image_file)
# 显示图像
image.show()
```
以上代码中,首先导入了PIL库中的Image模块。然后,通过指定图像文件的路径来选择要查看的图像文件。接下来,使用`Image.open()`函数打开图像文件,并将其赋值给一个变量image。最后,使用`image.show()`函数显示图像。
运行代码后,会弹出一个窗口显示图像。你可以在图像查看窗口中进行放大、缩小、旋转等操作。关闭图像查看窗口后,程序将继续执行后面的代码。
当然,这只是一个简单的图像查看器示例。你可以进一步扩展该程序,以添加更多的功能,比如缩放、裁剪、添加文本或标记物等。该程序还可以与其他库或模块进行结合,以实现更多的图像处理和操作。
### 回答3:
图像查看器是一种用于浏览和查看图像文件的应用程序。使用Python编写一个简单的图像查看器可以通过以下步骤实现:
1. 导入所需的库:在Python中,我们可以使用Pillow库来处理图像文件。首先,需要在代码开头导入Pillow库。
```python
from PIL import Image, ImageTk
import tkinter as tk
from tkinter import filedialog
```
2. 创建一个图形用户界面(GUI):使用Python的tkinter库创建一个基本的GUI窗口。
```python
window = tk.Tk()
window.title("图像查看器")
```
3. 定义打开图像的函数:创建一个函数来打开图像文件并在窗口中显示图像。
```python
def open_image():
filepath = filedialog.askopenfilename()
image = Image.open(filepath)
image = image.resize((400, 400)) # 调整图像大小以适应窗口
photo = ImageTk.PhotoImage(image)
image_label.configure(image=photo)
image_label.image = photo # 保留图像的引用
```
4. 创建一个按钮来选择图像文件:在窗口中添加一个按钮,当用户点击按钮时,调用打开图像的函数。
```python
open_button = tk.Button(window, text="打开图像", command=open_image)
open_button.pack()
```
5. 创建一个标签用于显示图像:在窗口中添加一个标签,用于显示打开的图像。
```python
image_label = tk.Label(window)
image_label.pack()
```
6. 运行应用程序:最后,使用tkinter库的mainloop()函数来运行图像查看器应用程序。
```python
window.mainloop()
```
通过执行以上代码,将会创建一个简单的图像查看器。用户可以点击按钮选择图像文件,然后在窗口中显示所选择的图像。
阅读全文
相关推荐
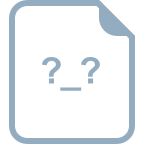
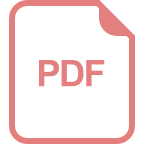















