import java.util.Scanner; public class Main { public static void main(String[] args) { Person p = new Person(); Scanner scanner = new Scanner(System.in); while (scanner.hasNextInt()) { int age = scanner.nextInt(); p.setAge(age); System.out.println(p.getAge()); } } } class Person { private int age; public int getAge(){ return age; } public void setAge(int age){ if(age > 200){ this.age = 200; }else if(age >= 0 && age <= 200){ this.age = age; }else if(age < 0){ this.age = 0; } } //write your code here...... }
时间: 2024-02-14 12:32:00 浏览: 34
你的代码是一个简单的Java程序,其中包含一个Main类和一个Person类。Main类中的main方法创建了一个Person对象和一个Scanner对象,然后进入一个循环,等待用户输入整数。每次输入一个整数后,调用Person对象的setAge方法来设置年龄,并通过调用getAge方法来获取并输出年龄。
你的问题是什么?我可以帮助你解决代码中的问题或提供其他方面的建议。
相关问题
构造Person类。包括姓名(name),性别(sex)和年龄(age)。提供所有属性的set和get函数,提供print函数打印其信息 输入描述: 姓名(name),性别(sex)和年龄(age) 输出描述: 用户信息 裁判测试程序样例: import java.util.Scanner; public class Main{ public static void main(String[] args) { Scanner scan = new Scanner(System.in); String name = scan.next(); String sex = scan.next(); int age = scan.nextInt(); Person p = new Person(); p.setName(name); p.setSex(sex); p.setAge(age); p.print(); scan.close(); } } /* 你的代码被嵌在这里 */
```
import java.util.Scanner;
class Person {
private String name;
private String sex;
private int age;
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getSex() {
return sex;
}
public void setAge(int age) {
this.age = age;
}
public int getAge() {
return age;
}
public void print() {
System.out.println("Name: " + name);
System.out.println("Sex: " + sex);
System.out.println("Age: " + age);
}
}
public class Main {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
String name = scan.next();
String sex = scan.next();
int age = scan.nextInt();
Person p = new Person();
p.setName(name);
p.setSex(sex);
p.setAge(age);
p.print();
scan.close();
}
}
```
import java.util.Scanner; public class Main{ public static void main(String[] args){ Scanner in = new Scanner(System.in); int age; age = in.nextInt(); Person p = new Person(age); age = in.nextInt(); try{ p.setAge(age); }catch(AgeException e){ } } } class Person{ int age; public Person(int age){ this.age = age; } public void setAge(int age) throws AgeException { if(this.age <=age){ this.age = age; }else{ throw new AgeException(); } } } class AgeException extends Exception{ }
这段代码是一个简单的 Java 程序,它通过输入年龄,并将年龄传递给 Person 类的对象 p,然后通过调用 setAge() 方法将年龄存储在 Person 对象中。如果输入的年龄小于对象中存储的年龄,则该方法会抛出一个 AgeException 异常。在主方法中,该异常被捕获并忽略了。该程序的作用是演示如何使用异常处理来验证输入的数据。
相关推荐
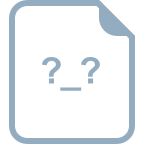












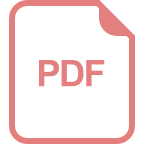