请用node.js 结合 axios封装get,post请求,传参url,及data,设置cookie,然后在前端js调用
时间: 2024-03-29 11:38:25 浏览: 17
好的,下面是封装get和post请求并设置cookie,以及在前端 JS 中调用的示例代码:
在后端 Node.js 中,我们可以按照之前的方式封装 get 和 post 请求,并且在实例中设置要发送的 cookie。这里提供一个封装后端 API 的示例代码:
```javascript
const axios = require('axios');
const instance = axios.create({
baseURL: 'https://example.com',
timeout: 5000,
headers: {
'Content-Type': 'application/json',
'Accept': 'application/json'
}
});
instance.defaults.headers.common['Cookie'] = `cookieName=cookieValue`;
// 封装 GET 请求
function get(url, params) {
return instance.get(url, { params });
}
// 封装 POST 请求
function post(url, data) {
return instance.post(url, data);
}
module.exports = {
get,
post
};
```
在前端 JS 中,我们可以使用 Ajax 或 fetch 等方式调用后端 Node.js API,获取数据或提交数据。这里提供一个使用 jQuery Ajax 调用后端 API 的示例代码:
```javascript
$.ajax({
url: 'https://example.com/api/data',
type: 'GET',
dataType: 'json',
xhrFields: {
withCredentials: true
},
success: function(data) {
console.log(data);
},
error: function(xhr, status, error) {
console.error(error);
}
});
$.ajax({
url: 'https://example.com/api/data',
type: 'POST',
dataType: 'json',
xhrFields: {
withCredentials: true
},
data: {
name: 'John',
age: 30
},
success: function(data) {
console.log(data);
},
error: function(xhr, status, error) {
console.error(error);
}
});
```
在上面的代码中,我们使用了 jQuery 的 `$.ajax()` 方法发送 GET 和 POST 请求,并且设置了 `xhrFields` 选项,让 Ajax 能够携带跨域请求中的 Cookie。在请求成功时,我们通过 `success` 回调函数获取服务器返回的数据,在请求失败时,我们通过 `error` 回调函数获取错误信息。
相关推荐
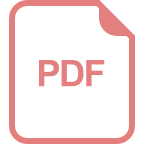
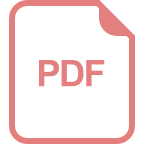














