用python写一个接口性能测试
时间: 2023-08-31 12:38:54 浏览: 126
### 回答1:
好的,以下是用Python编写一个接口性能测试的示例代码:
```python
import requests
import time
def test_api(url, num_requests):
start_time = time.time()
for i in range(num_requests):
response = requests.get(url)
print(f"Request {i+1} - Status code: {response.status_code}")
end_time = time.time()
total_time = end_time - start_time
print(f"Total time: {total_time} seconds")
print(f"Average time per request: {total_time/num_requests} seconds")
if __name__ == '__main__':
test_api('https://jsonplaceholder.typicode.com/posts/1', 10)
```
这个示例代码通过requests库发送GET请求来测试API接口的性能。在`test_api`函数中,我们使用`start_time`变量记录测试开始时间,使用循环发送指定数量的请求,然后使用`end_time`变量记录测试结束时间。最后,我们计算测试总共耗时和每个请求的平均耗时,并打印输出。
你可以将`test_api`函数的参数修改为你需要测试的API接口的URL和请求次数,然后运行代码进行测试。
### 回答2:
接口性能测试是用于评估系统接口性能和稳定性的一种测试方法。使用Python编写的接口性能测试脚本可以使用多种方式进行,下面是一个示例:
```python
import requests
import time
# 定义接口性能测试函数
def test_interface_performance(url):
start_time = time.time() # 记录测试开始时间
response = requests.get(url) # 发送接口请求
end_time = time.time() # 记录测试结束时间
# 计算接口请求响应时间
response_time = end_time - start_time
# 输出接口请求结果和响应时间
print("接口请求结果:", response.status_code)
print("接口响应时间:", response_time)
# 测试接口性能
test_interface_performance("http://example.com/api")
```
上述代码使用了Python的`requests`库发送接口请求,并使用`time`库记录测试开始和结束时间,从而计算接口请求的响应时间。通过调用`test_interface_performance`函数,并传入待测试的接口URL,即可进行接口性能测试。
这只是一个简单示例,实际的接口性能测试可能还需要更多的功能和处理逻辑,例如并发测试、数据统计和结果可视化等,但以上代码提供了一个基本的框架来进行接口性能测试。
### 回答3:
编写一个接口性能测试的Python脚本可以通过使用Python的requests和time模块来实现。以下是一个示例代码:
```python
import requests
import time
def api_performance_test(url):
start_time = time.time()
response = requests.get(url) # 发送GET请求
end_time = time.time()
latency = end_time - start_time # 计算接口请求延迟时间
response_time = response.elapsed.total_seconds() # 获取服务器响应时间
status_code = response.status_code # 获取服务器返回的状态码
print("接口请求延迟时间: %.2f秒" % latency)
print("服务器响应时间: %.2f秒" % response_time)
print("服务器返回状态码: %d" % status_code)
# 调用示例
api_performance_test("http://www.example.com/api")
```
以上代码通过发送GET请求,计算接口请求延迟时间和服务器响应时间,并打印出结果。在实际使用时,你可以根据需要修改和补充代码部分,以适应具体的接口性能测试需求。
相关推荐
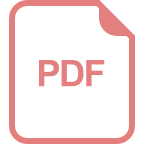
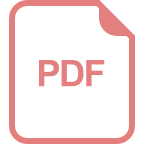
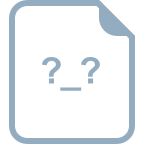







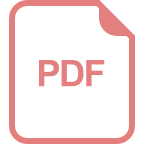
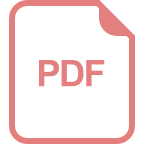
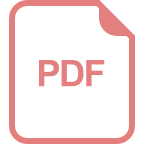
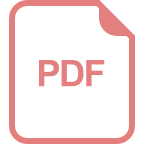
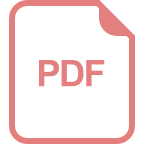
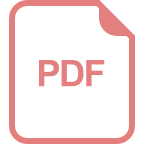
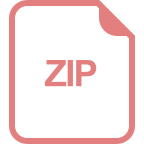
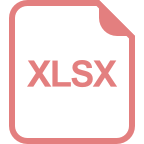