app实现调用aidl接口
时间: 2024-01-10 21:02:36 浏览: 27
1. 创建aidl文件
在app/src/main/aidl目录下创建一个名为IHelloWorld.aidl的文件,内容如下:
```
package com.example.helloworld;
interface IHelloWorld {
String sayHello();
void setHello(String hello);
}
```
2. 实现aidl接口
在app/src/main/java目录下创建一个名为HelloWorldService的Java类,实现IHelloWorld接口,代码如下:
```
package com.example.helloworld;
import android.app.Service;
import android.content.Intent;
import android.os.IBinder;
import android.os.RemoteException;
public class HelloWorldService extends Service {
private String mHello = "Hello World!";
private final IHelloWorld.Stub mBinder = new IHelloWorld.Stub() {
@Override
public String sayHello() throws RemoteException {
return mHello;
}
@Override
public void setHello(String hello) throws RemoteException {
mHello = hello;
}
};
@Override
public IBinder onBind(Intent intent) {
return mBinder;
}
}
```
3. 注册服务
在app/src/main/AndroidManifest.xml文件中注册服务,代码如下:
```
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.helloworld">
<application
android:allowBackup="true"
android:label="@string/app_name"
android:icon="@mipmap/ic_launcher"
android:theme="@style/AppTheme">
<service android:name=".HelloWorldService"
android:exported="true">
<intent-filter>
<action android:name="com.example.helloworld.IHelloWorld" />
</intent-filter>
</service>
</application>
</manifest>
```
4. 调用aidl接口
在app/src/main/java目录下创建一个名为MainActivity的Java类,代码如下:
```
package com.example.helloworld;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.os.IBinder;
import android.os.RemoteException;
import android.util.Log;
public class MainActivity extends AppCompatActivity {
private static final String TAG = "MainActivity";
private IHelloWorld mHelloWorld;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
IBinder binder = getService("com.example.helloworld.IHelloWorld");
mHelloWorld = IHelloWorld.Stub.asInterface(binder);
try {
String hello = mHelloWorld.sayHello();
Log.d(TAG, "sayHello: " + hello);
mHelloWorld.setHello("Hello Android!");
hello = mHelloWorld.sayHello();
Log.d(TAG, "sayHello: " + hello);
} catch (RemoteException e) {
e.printStackTrace();
}
}
private IBinder getService(String serviceName) {
IBinder binder = null;
Intent intent = new Intent(serviceName);
intent.setPackage(getPackageName());
ServiceConnection conn = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName name, IBinder service) {
binder = service;
}
@Override
public void onServiceDisconnected(ComponentName name) {
}
};
bindService(intent, conn, Context.BIND_AUTO_CREATE);
return binder;
}
}
```
运行app,查看Logcat中的输出信息,即可看到调用aidl接口的结果。
相关推荐
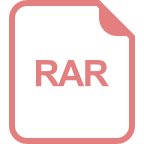
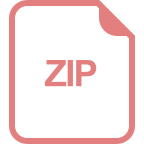
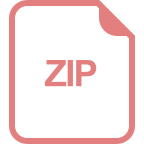















