从键盘输入任意字符串,将其中的所有大写字母改成小写字母,而所有小写字母改成大写字母,其他字符不变
时间: 2024-05-09 11:21:48 浏览: 14
好的,您可以使用以下Python代码来实现这个功能:
```python
string = input("请输入要进行大小写转换的字符串:") # 从键盘输入字符串
result = "" # 存储转换后的结果
# 遍历字符串中的每个字符
for c in string:
# 判断字符的大小写,并进行转换
if c.isupper(): # 大写字母转小写字母
result += c.lower()
elif c.islower(): # 小写字母转大写字母
result += c.upper()
else: # 其他字符不变
result += c
print("转换后的字符串为:", result) # 输出转换后的结果
```
您可以将上述代码复制到您的Python编辑器中运行,并根据提示输入要进行大小写转换的字符串,最终会输出转换后的结果。
相关问题
从键盘输入任意字符串,将其中的所有大写字母改成小写字母,而所有小写字母改成大写字母,其他字符不变,用C语言代码
以下是代码示例:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
printf("请输入字符串:");
fgets(str, sizeof(str), stdin);
for (int i = 0; str[i] != '\0'; i++) {
if (isupper(str[i])) {
str[i] = tolower(str[i]);
} else if (islower(str[i])) {
str[i] = toupper(str[i]);
}
}
printf("输出结果:%s\n", str);
return 0;
}
```
使用 isupper() 函数判断是否为大写字母,使用 tolower() 函数将大写字母转换成小写字母,同理,使用 islower() 函数判断是否为小写字母,使用 toupper() 函数将小写字母转换成大写字母。最后输出转换后的字符串即可。
从键盘输入一个字符串,将其中的大写字母变成小写字母,其它字符不变 。
可以用Python实现这个功能,代码如下:
```python
string = input("请输入一个字符串:")
new_string = ""
for char in string:
if char.isupper():
new_string += char.lower()
else:
new_string += char
print("转换后的字符串为:", new_string)
```
代码中,我们先通过`input()`函数获取用户输入的字符串,并将其保存到变量`string`中。
然后声明一个新的空字符串`new_string`,用来存储转换后的结果。
接着通过`for`循环遍历原始字符串中的每一个字符,如果该字符是大写字母,则将其转换为小写字母后添加到`new_string`中,否则直接将该字符添加到`new_string`中。
最后输出转换后的结果即可。
相关推荐
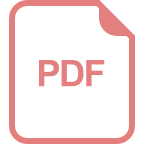
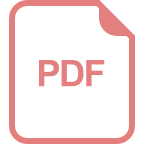
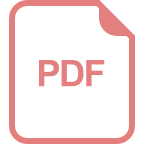
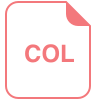











