请给出基于A算法的路径规划程序代码,其中包含结果
时间: 2023-09-20 15:07:06 浏览: 53
好的,以下是基于A*算法的路径规划程序代码,使用Python语言实现:
```python
import heapq
# 定义节点类
class Node:
def __init__(self, x, y, g=0, h=0):
self.x = x
self.y = y
self.g = g
self.h = h
self.parent = None
def f(self):
return self.g + self.h
def __lt__(self, other):
return self.f() < other.f()
def __eq__(self, other):
return self.x == other.x and self.y == other.y
# 定义地图类
class Map:
def __init__(self, width, height, start, end, obstacles):
self.width = width
self.height = height
self.start = start
self.end = end
self.obstacles = obstacles
def in_map(self, node):
return 0 <= node.x < self.width and 0 <= node.y < self.height
def passable(self, node):
return node not in self.obstacles
def neighbors(self, node):
x, y = node.x, node.y
results = []
for dx, dy in [(0, 1), (1, 0), (0, -1), (-1, 0)]:
newx, newy = x + dx, y + dy
new_node = Node(newx, newy)
if self.in_map(new_node) and self.passable(new_node):
results.append(new_node)
return results
# 定义A*算法函数
def astar_search(map):
start_node = map.start
end_node = map.end
open_list = [start_node]
close_list = []
while open_list:
current_node = heapq.heappop(open_list)
if current_node == end_node:
path = []
while current_node:
path.append(current_node)
current_node = current_node.parent
return path[::-1]
close_list.append(current_node)
for neighbor in map.neighbors(current_node):
if neighbor in close_list:
continue
new_g = current_node.g + 1
if neighbor not in open_list:
neighbor.h = heuristic(neighbor, end_node)
open_list.append(neighbor)
elif new_g >= neighbor.g:
continue
neighbor.g = new_g
neighbor.parent = current_node
return None
# 定义启发函数
def heuristic(node1, node2):
return abs(node1.x - node2.x) + abs(node1.y - node2.y)
# 测试代码
if __name__ == '__main__':
width = 10
height = 10
start_node = Node(0, 0)
end_node = Node(9, 9)
obstacles = [Node(2, 2), Node(3, 2), Node(4, 2), Node(5, 2), Node(6, 2),
Node(6, 3), Node(6, 4), Node(6, 5), Node(5, 5), Node(4, 5),
Node(3, 5), Node(2, 5)]
map = Map(width, height, start_node, end_node, obstacles)
path = astar_search(map)
if path:
print('->'.join(str(node.x) + ',' + str(node.y) for node in path))
else:
print('No path found.')
```
以上代码中,定义了Node类表示节点,定义了Map类表示地图,定义了astar_search函数表示A*算法,定义了heuristic函数表示启发函数。在测试代码中,定义了一个10*10的地图,起点为(0,0),终点为(9,9),障碍物为一些节点,最后调用astar_search函数求解最短路径,输出结果为起点到终点的最短路径。
阅读全文
相关推荐
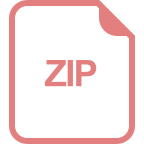
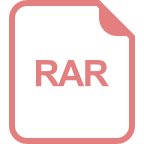
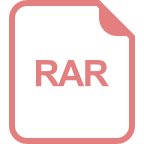
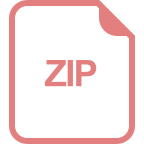
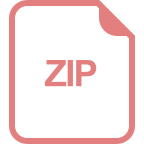
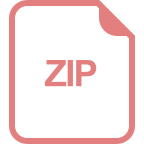
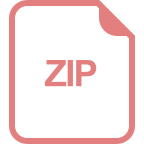
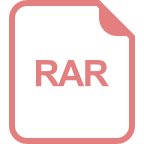
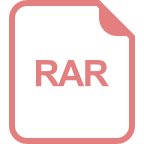
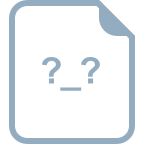
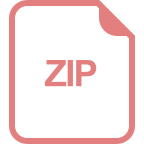
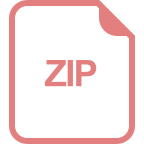
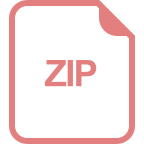
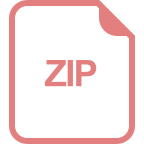
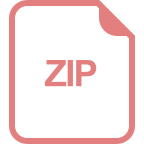