编写一个程序,用来分别求3个整数、3个双精度数的最大.值,要求使用函数重载来完成。
时间: 2023-05-21 11:05:28 浏览: 62
以下是一个函数重载的示例程序,用于分别找到三个整数和三个双精度数的最大值:
#include <iostream>
using namespace std;
int max(int a, int b, int c) {
int max_value = a;
if (b > max_value) {
max_value = b;
}
if (c > max_value) {
max_value = c;
}
return max_value;
}
double max(double a, double b, double c) {
double max_value = a;
if (b > max_value) {
max_value = b;
}
if (c > max_value) {
max_value = c;
}
return max_value;
}
int main() {
int int_max = max(1, 2, 3);
double double_max = max(1.1, 2.2, 3.3);
cout << "最大值(整数):" << int_max << endl;
cout << "最大值(双精度数):" << double_max << endl;
return 0;
}
关于 "lua closure factory 完整代码" 的问题,这是关于 Lua 编程的一个问题。下面是一个简单的示例程序,它演示了如何使用闭包工厂函数:
function newCounter()
local count = 0
return function()
count = count + 1
return count
end
end
c1 = newCounter()
print(c1()) -- 输出1
print(c1()) -- 输出2
c2 = newCounter()
print(c2()) -- 输出1
print(c1()) -- 输出3
print(c2()) -- 输出2
关于 "中文加密" 的问题,我认为你可能指的是中文字符串的加密算法。下面是使用简单的替换算法对中文字符串进行加密/解密的示例程序:
#include <iostream>
#include <string>
using namespace std;
string encrypt(string text) {
for (int i = 0; i < text.size(); i++) {
text[i] += 1; // 将每个字符替换为其后一个字符
}
return text;
}
string decrypt(string text) {
for (int i = 0; i < text.size(); i++) {
text[i] -= 1; // 将每个字符替换为其前一个字符
}
return text;
}
int main() {
string text = "中国加密"; // 原始字符串
cout << "原文:" << text << endl;
string encrypted_text = encrypt(text); // 加密后的字符串
cout << "加密后:" << encrypted_text << endl;
string decrypted_text = decrypt(encrypted_text); // 解密后的字符串
cout << "解密后:" << decrypted_text << endl;
return 0;
}
当然,这只是一个简单的示例程序,实际中使用的加密算法应该更加复杂和安全。
相关推荐
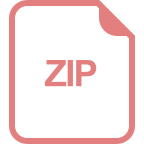
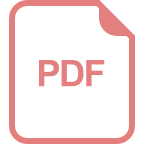














