Complete the Mint and Coin classes so that the coins created by a mint have the correct year and worth. Each Mint instance has a year stamp. The update method sets the year stamp to the current_year class attribute of the Mint class. The create method takes a subclass of Coin and returns an instance of that class stamped with the mint's year (which may be different from Mint.current_year if it has not been updated.) A Coin's worth method returns the cents value of the coin plus one extra cent for each year of age beyond 50. A coin's age can be determined by subtracting the coin's year from the current_year class attribute of the Mint class.
时间: 2024-02-29 11:53:13 浏览: 19
Here's an implementation of the Mint and Coin classes with the required functionality:
```python
class Mint:
current_year = 2021
def __init__(self, year):
self.year = year
def update(self):
self.year = Mint.current_year
def create(self, coin_class):
return coin_class(self.year)
class Coin:
worth_in_cents = 0
def __init__(self, year):
self.year = year
def age(self):
return Mint.current_year - self.year
def worth(self):
extra_cents = max(0, self.age() - 50)
return Coin.worth_in_cents + extra_cents
class Quarter(Coin):
worth_in_cents = 25
class Dime(Coin):
worth_in_cents = 10
class Nickel(Coin):
worth_in_cents = 5
class Penny(Coin):
worth_in_cents = 1
```
In this implementation, the `Mint` class has an instance variable `year` which represents the year stamp of the mint. The `update` method sets this year stamp to the current year (`Mint.current_year`).
The `create` method takes a coin class as an argument and returns an instance of that class stamped with the mint's year. This is achieved by passing the mint's year to the constructor of the coin class.
The `Coin` class has an instance variable `year` which represents the year stamp of the coin. The `age` method calculates the age of the coin by subtracting the coin's year from the current year of the mint (`Mint.current_year`). The `worth` method calculates the worth of the coin by adding the base worth (stored in the `worth_in_cents` class variable) to one extra cent for each year of age beyond 50.
Finally, there are subclasses of `Coin` for each denomination, with the `worth_in_cents` class variable set appropriately.
相关推荐
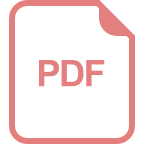
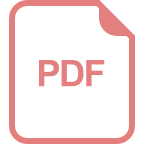
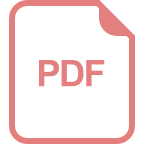














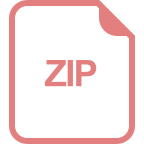