关于室内消防安全与急救的Python代码
时间: 2023-11-05 16:55:02 浏览: 107
以下是一个简单的Python代码,用于模拟室内火灾的情况,并提供基本的急救指南:
```python
import time
# 定义一个人员类
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
self.is_alive = True
self.is_injured = False
def __repr__(self):
return self.name
# 定义一个火灾类
class Fire:
def __init__(self, location):
self.location = location
self.is_active = True
def __repr__(self):
return f"Fire at {self.location}"
# 定义一个急救类
class FirstAid:
def __init__(self):
self.instructions = {
"burns": "Run cool water over the burn for 10-15 minutes. Cover with a sterile gauze bandage.",
"smoke inhalation": "Get the person to fresh air right away. If breathing has stopped, perform CPR. Call for emergency medical help.",
"cuts": "Apply pressure with a clean cloth or bandage for several minutes to stop bleeding. If bleeding doesn’t stop after 10 minutes, seek medical advice.",
"heart attack": "Call for emergency medical help. Ask the person to chew and swallow an aspirin if they are able to. Wait for the ambulance.",
}
def provide_instructions(self, injury):
return self.instructions.get(injury, "No instructions available for this injury.")
def main():
# 定义人员和火灾位置
john = Person("John", 35)
sarah = Person("Sarah", 25)
fire = Fire("Kitchen")
# 模拟火灾
print(f"{fire} has started!")
time.sleep(2)
# John和Sarah尝试逃离火灾
for person in [john, sarah]:
if person.is_alive:
print(f"{person} is trying to escape!")
time.sleep(2)
# 判断人员是否受伤
if fire.is_active:
person.is_injured = True
print(f"{person} has been injured!")
time.sleep(2)
# 提供急救指导
first_aid = FirstAid()
print(first_aid.provide_instructions("smoke inhalation"))
time.sleep(2)
# 判断人员是否幸存
if person.is_injured:
person.is_alive = False
print(f"{person} did not survive.")
time.sleep(2)
else:
print(f"{person} has escaped safely!")
time.sleep(2)
# 判断火灾是否熄灭
if fire.is_active:
print(f"{fire} is still burning! Call the fire department!")
else:
print(f"{fire} has been extinguished. The situation is under control.")
if __name__ == "__main__":
main()
```
这个示例代码模拟了一个火灾情况,并提供了基本的急救指南。当火灾发生时,程序模拟两个人员(John和Sarah)试图逃离火灾。如果一个人员受伤,程序会提供相应的急救指导。最后,程序会判断火灾是否熄灭,以及人员是否幸存。请注意,这只是一个简单的模拟,仅作为了解如何使用Python进行这种类型的模拟的示例。
阅读全文
相关推荐
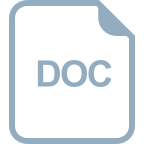
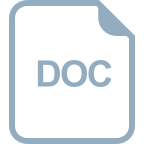
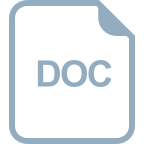
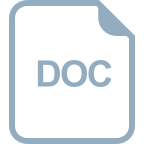
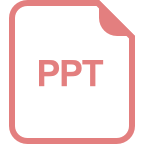
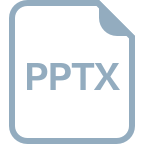
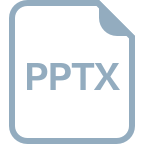
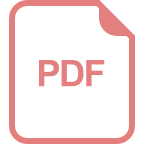
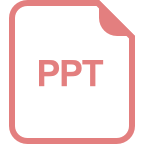
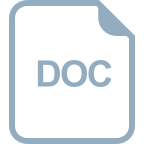
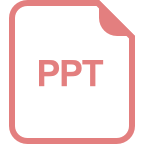
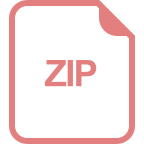
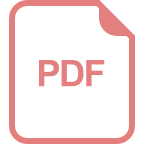
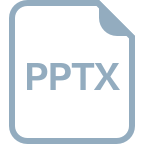
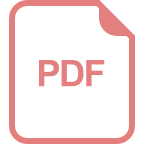
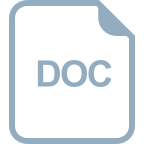
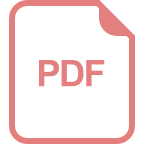
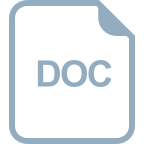
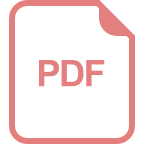