SPI读写flash驱动程序解释
时间: 2023-09-15 12:22:18 浏览: 65
SPI读写flash驱动程序是一种用于与SPI接口器件通信的驱动程序。该驱动程序可以实现对SPI接口的flash存储器进行读写操作,包括写入数据到flash存储器和从flash存储器读取数据。具体的实现过程包括以下几个步骤:
1. 初始化SPI接口:在使用SPI接口进行通信之前,需要对SPI接口进行初始化,包括设置时钟频率、数据位数、传输模式等参数。
2. 选择flash存储器:使用SPI接口进行通信时,需要先选择要通信的SPI器件,这里选择flash存储器。
3. 发送命令:对于不同的操作,需要发送不同的命令码,比如写入数据、读取数据等。
4. 发送数据:在进行写入操作时,需要向flash存储器中写入数据,这里需要将数据通过SPI接口发送给flash存储器。
5. 读取数据:在进行读取操作时,需要从flash存储器中读取数据,这里需要通过SPI接口从flash存储器中读取数据。
6. 取消选择器件:完成操作后,需要取消选择SPI器件,释放SPI总线。
通过以上步骤,可以实现对SPI接口的flash存储器进行读写操作。同时,SPI读写flash驱动程序还需要考虑数据传输的稳定性和可靠性,以及对错误的处理和异常情况的处理。
相关问题
spi flash读写驱动程序实例
以下是一个简单的SPI Flash读写驱动程序示例,使用的是Linux内核自带的SPI驱动接口。
#include <linux/module.h>
#include <linux/spi/spi.h>
#include <linux/mtd/mtd.h>
#include <linux/mtd/partitions.h>
#define FLASH_PAGE_SIZE 256
#define FLASH_SECTOR_SIZE 4096
#define FLASH_BLOCK_SIZE 65536
struct spi_flash {
struct mtd_info mtd;
struct spi_device *spi;
};
static int spi_flash_probe(struct spi_device *spi)
{
struct spi_flash *flash;
struct mtd_partition *parts;
int nparts, err;
flash = kzalloc(sizeof(struct spi_flash), GFP_KERNEL);
if (!flash) {
dev_err(&spi->dev, "Failed to allocate memory for spi_flash\n");
return -ENOMEM;
}
flash->spi = spi;
/* Set up MTD structure */
flash->mtd.name = spi->modalias;
flash->mtd.owner = THIS_MODULE;
flash->mtd.type = MTD_NORFLASH;
flash->mtd.flags = MTD_CAP_NORFLASH;
flash->mtd.erasesize = FLASH_BLOCK_SIZE;
flash->mtd.writesize = FLASH_PAGE_SIZE;
flash->mtd.writebufsize = FLASH_PAGE_SIZE;
/* Register MTD device */
err = mtd_device_register(&flash->mtd, NULL, 0);
if (err) {
dev_err(&spi->dev, "Failed to register MTD device, error %d\n", err);
kfree(flash);
return err;
}
/* Set up partition table */
nparts = get_mtd_device_partitions(&flash->mtd, &parts, 0);
if (nparts <= 0) {
dev_err(&spi->dev, "Failed to create partition table\n");
mtd_device_unregister(&flash->mtd);
kfree(flash);
return -EINVAL;
}
/* Print information about the device */
dev_info(&spi->dev, "SPI Flash device detected, %d partitions.\n", nparts);
dev_info(&spi->dev, "Flash device size %llu bytes, erase size %d bytes, write size %d bytes.\n",
(unsigned long long)flash->mtd.size, flash->mtd.erasesize, flash->mtd.writesize);
return 0;
}
static int spi_flash_remove(struct spi_device *spi)
{
struct spi_flash *flash = spi_get_drvdata(spi);
mtd_device_unregister(&flash->mtd);
kfree(flash);
return 0;
}
static const struct of_device_id spi_flash_of_match[] = {
{ .compatible = "spansion, s25fl064k", },
{ /* end of table */ }
};
MODULE_DEVICE_TABLE(of, spi_flash_of_match);
static struct spi_driver spi_flash_driver = {
.driver = {
.name = "spi_flash",
.owner = THIS_MODULE,
.of_match_table = spi_flash_of_match,
},
.probe = spi_flash_probe,
.remove = spi_flash_remove,
};
static int __init spi_flash_init(void)
{
return spi_register_driver(&spi_flash_driver);
}
static void __exit spi_flash_exit(void)
{
spi_unregister_driver(&spi_flash_driver);
}
module_init(spi_flash_init);
module_exit(spi_flash_exit);
MODULE_LICENSE("GPL");
MODULE_AUTHOR("Your Name");
MODULE_DESCRIPTION("SPI Flash driver");
MODULE_ALIAS("spi:spi_flash");
以上代码定义了一个名为spi_flash的驱动程序,它基于Linux内核自带的SPI驱动接口,并提供了一个MTD设备,可以进行SPI Flash的读写操作。在probe函数中,驱动程序会初始化MTD设备,并创建分区表。在remove函数中,驱动程序会注销MTD设备。在spi_flash_init函数中,驱动程序会注册SPI驱动程序。在spi_flash_exit函数中,驱动程序会注销SPI驱动程序。
spi flash读写驱动框架
SPI Flash读写驱动框架是一个用于编写SPI Flash驱动程序的基本框架。它通常由以下几个部分组成:
1. 硬件抽象层:该层提供与具体硬件相关的函数和接口,例如SPI总线控制器的驱动程序和GPIO控制器的驱动程序。该层的主要目的是隐藏硬件细节并提供统一的接口。
2. Flash芯片抽象层:该层提供与Flash芯片相关的函数和接口,例如读写Flash数据、擦除Flash数据、检查Flash状态等。该层的主要目的是隐藏Flash芯片的底层细节并提供统一的接口。
3. 文件系统层:该层提供与文件系统相关的函数和接口,例如打开文件、读取文件、写入文件等。该层的主要目的是提供一个高层次的接口,使应用程序能够方便地读写Flash存储器。
4. 应用程序层:该层是最高层,提供与具体应用程序相关的函数和接口。例如,如果应用程序需要从Flash存储器中读取配置文件,则该层将提供读取文件、解析文件等功能。
SPI Flash读写驱动框架的主要优点是它提供了一种结构化的方法来编写Flash驱动程序,使得开发人员能够更容易地实现和维护这些程序。此外,该框架还提供了可移植性,使得驱动程序可以在不同的硬件平台上运行。
相关推荐
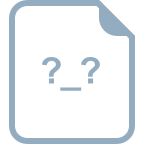
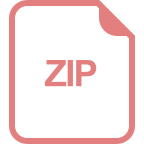












