python实现glove
时间: 2023-12-04 15:57:08 浏览: 74
GloVe(Global Vectors for Word Representation)是一种用于生成词向量表示的算法。它结合了全局词汇统计信息和局部上下文窗口中的词共现计数信息。下面是python实现glove算法的基本步骤:
1. 导入所需的库
```python
import numpy as np
from collections import Counter
```
2. 定义函数来计算共现矩阵
```python
def co_occurrence_matrix(corpus, window_size):
words = corpus.split()
word_freq = dict(Counter(words))
vocab = list(word_freq.keys())
vocab_size = len(vocab)
co_matrix = np.zeros((vocab_size, vocab_size), dtype=np.int32)
for i in range(len(words)):
w_i = words[i]
for j in range(i - window_size, i + window_size + 1):
if j >= 0 and j < len(words) and j != i:
w_j = words[j]
co_matrix[vocab.index(w_i), vocab.index(w_j)] += 1
return co_matrix, vocab
```
3. 定义函数来计算GloVe矩阵
```python
def glove_matrix(co_matrix, embedding_dim=50, learning_rate=0.05, epochs=100):
np.random.seed(0)
W = np.random.uniform(-0.5, 0.5, (co_matrix.shape[0], embedding_dim))
b = np.random.uniform(-0.5, 0.5, co_matrix.shape[0])
x_max = 100
alpha = 0.75
p_i = np.sum(co_matrix, axis=1) / np.sum(co_matrix)
log_co_matrix = np.log(co_matrix + 1)
for epoch in range(epochs):
f_w = np.zeros_like(co_matrix, dtype=np.float32)
for i in range(co_matrix.shape[0]):
for j in range(co_matrix.shape[1]):
if co_matrix[i][j] > 0:
w_ij = np.dot(W[i], W[j]) + b[i] + b[j]
f_wij = (co_matrix[i][j] / x_max) ** alpha if co_matrix[i][j] < x_max else 1
f_w[i][j] = f_wij * w_ij
grad_w = np.zeros_like(W, dtype=np.float32)
grad_b = np.zeros_like(b, dtype=np.float32)
for i in range(co_matrix.shape[0]):
for j in range(co_matrix.shape[1]):
if co_matrix[i][j] > 0:
w_ij = np.dot(W[i], W[j]) + b[i] + b[j]
f_wij = (co_matrix[i][j] / x_max) ** alpha if co_matrix[i][j] < x_max else 1
delta = f_wij * (w_ij - np.log(co_matrix[i][j]))
grad_w[i] += delta * W[j]
grad_w[j] += delta * W[i]
grad_b[i] += delta
grad_b[j] += delta
W -= learning_rate * grad_w
b -= learning_rate * grad_b
return W
```
4. 使用函数来计算词向量
```python
corpus = "apple banana orange apple apple banana"
co_matrix, vocab = co_occurrence_matrix(corpus, window_size=2)
W = glove_matrix(co_matrix, embedding_dim=50, learning_rate=0.05, epochs=100)
word_to_index = {word: i for i, word in enumerate(vocab)}
index_to_word = {i: word for i, word in enumerate(vocab)}
word_vecs = {}
for word, i in word_to_index.items():
word_vecs[word] = W[i]
```
这样,我们就可以得到一个包含每个单词词向量的字典。
阅读全文
相关推荐
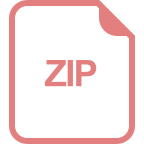
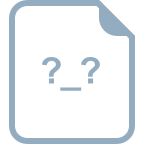
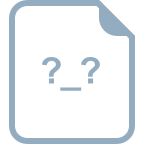


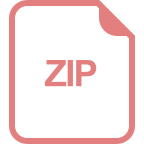
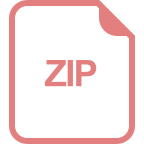
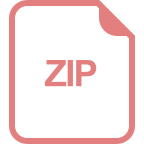
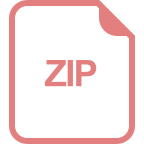


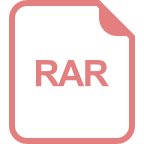