react项目怎么重新渲染当前页面,具体的几个示例代码
时间: 2023-12-10 09:37:08 浏览: 26
在 React 中重新渲染当前页面有几种方式,下面是几个示例代码:
1. 使用 setState 方法:
```
class App extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
this.setState({
count: this.state.count + 1
});
}
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.handleClick}>Click me</button>
</div>
);
}
}
```
当点击按钮时,会调用 `handleClick` 方法并更新状态,从而重新渲染当前页面。
2. 使用 forceUpdate 方法:
```
class App extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
this.setState({
count: this.state.count + 1
});
this.forceUpdate();
}
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.handleClick}>Click me</button>
</div>
);
}
}
```
当点击按钮时,除了更新状态外,还会调用 `forceUpdate` 方法强制重新渲染当前页面。
3. 使用 key 属性:
```
class App extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
this.setState({
count: this.state.count + 1
});
}
render() {
return (
<div key={this.state.count}>
<p>Count: {this.state.count}</p>
<button onClick={this.handleClick}>Click me</button>
</div>
);
}
}
```
当状态更新时,重新渲染当前页面的关键在于 `key` 属性的变化,而不是直接重新渲染整个组件。因此,在组件的根元素中设置 `key` 属性并将其设置为状态的值,可以实现重新渲染当前页面的效果。
相关推荐
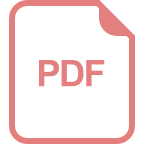
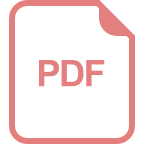














