全排列next_permutation
时间: 2023-04-25 22:03:28 浏览: 124
next_permutation是一个C++ STL库中的函数,用于生成下一个排列。它接受一个迭代器作为参数,并将该迭代器指向的序列转换为下一个排列。如果没有下一个排列,则返回false。如果存在下一个排列,则返回true。
相关问题
c++next_permutation全排列结构体
`std::next_permutation`是C++标准库中的一个算法,它用于找到一个容器(通常是`std::vector`或`std::array`)中元素的下一个排列。这个函数在排序后的序列上工作,如果当前排列已经是最大的,则返回`false`;否则,它会找到并返回下一个排列,使得第一个比第二个大,第二个比第三个大,以此类推。
在C++中,`next_permutation`通常与`std::algorithm`头文件一起使用,其原型如下:
```cpp
template <class BidirectionalIterator>
bool next_permutation(BidirectionalIterator first, BidirectionalIterator last);
```
参数:
- `first`:一个迭代器,指向序列的第一个元素。
- `last`:一个迭代器,指向序列的下一个位置(不包括)。
例如,你可以这样使用这个函数:
```cpp
#include <algorithm>
#include <vector>
std::vector<int> arr = {1, 2, 3};
bool is_sorted = std::is_sorted(arr.begin(), arr.end()); // 假设arr已排序
if (!is_sorted) {
do {
// 打印当前排列
for (const auto &val : arr) {
std::cout << val << " ";
}
std::cout << "\n";
// 接下来获取下一个排列
std::next_permutation(arr.begin(), arr.end());
} while (std::next_permutation(arr.begin(), arr.end()));
}
```
next_permutation函数解决全排列问题
next_permutation函数是C++标准库中的一个函数,用于解决全排列问题。它的作用是将一个序列重新排列为下一个字典序更大的排列。
使用next_permutation函数,你可以逐步生成序列的所有不同排列。它接受两个迭代器作为参数,表示序列的起始和结束位置。函数会将序列重新排列为下一个字典序更大的排列,并返回一个布尔值,表示是否成功生成了下一个排列。
下面是一个使用next_permutation函数解决全排列问题的示例代码:
```cpp
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3};
std::sort(nums.begin(), nums.end()); // 需要先将序列排序
do {
// 处理当前排列
for (int num : nums) {
std::cout << num << " ";
}
std::cout << std::endl;
} while (std::next_permutation(nums.begin(), nums.end()));
return 0;
}
```
在这个示例中,我们定义了一个包含数字1、2、3的vector。使用std::sort函数将序列排序,然后使用do-while循环和std::next_permutation函数逐步生成并处理所有不同的排列。
输出结果为:
```
1 2 3
1 3 2
2 1 3
2 3 1
3 1 2
3 2 1
```
这样,我们就可以使用next_permutation函数解决全排列问题了。希望对你有帮助!如有其他问题,请继续提问。
阅读全文
相关推荐
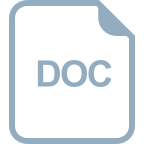
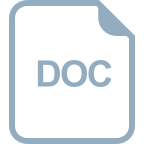
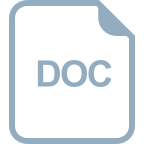
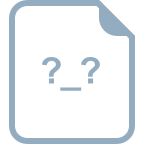
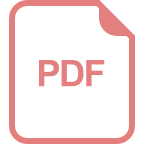










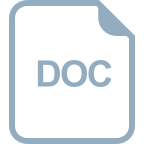