dbscan聚类五分类读取数据代码实现
时间: 2023-08-03 22:09:06 浏览: 148
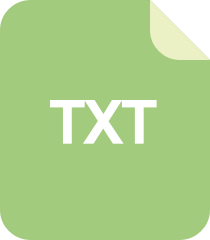
DBSCAN聚类算法实现代码
Sure! Here's an example code for using DBSCAN clustering algorithm for five-class classification and reading data in Python:
```python
import pandas as pd
from sklearn.cluster import DBSCAN
from sklearn.preprocessing import StandardScaler
# Read the data from a CSV file
data = pd.read_csv('data.csv')
# Separate the features and labels
X = data.drop('label', axis=1) # Features
y = data['label'] # Labels
# Perform feature scaling
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
# Initialize and fit the DBSCAN model
dbscan = DBSCAN(eps=0.5, min_samples=5)
dbscan.fit(X_scaled)
# Get the predicted labels
predicted_labels = dbscan.labels_
# Assign a class label to each cluster
cluster_labels = []
unique_labels = set(predicted_labels)
for label in unique_labels:
if label == -1:
cluster_labels.append('Noise')
else:
cluster_labels.append('Class ' + str(label))
# Print the assigned labels for each data point
for i in range(len(X)):
print('Data point {}: Predicted label: {}'.format(i, cluster_labels[predicted_labels[i]]))
```
In this code, we assume that the data is stored in a CSV file named `data.csv`. You need to modify the file path according to your own data.
First, we import the necessary libraries, including `pandas` for reading the CSV file, `DBSCAN` class from `sklearn.cluster` for implementing DBSCAN clustering, and `StandardScaler` from `sklearn.preprocessing` for feature scaling.
Then, we read the data from the CSV file and separate the features (X) and labels (y). We perform feature scaling using `StandardScaler` to standardize the features.
Next, we initialize a DBSCAN object with the desired parameters such as `eps` (the maximum distance between two samples to be considered in the same neighborhood) and `min_samples` (the minimum number of samples in a neighborhood for a point to be considered as a core point). We fit the DBSCAN model to the scaled features.
After that, we get the predicted labels for each data point using `dbscan.labels_`. We assign a class label to each cluster, and for points classified as noise (label -1), we assign the label 'Noise'.
Finally, we print the assigned labels for each data point.
Remember to replace `'data.csv'` with the actual path to your data file, and modify the parameters of DBSCAN according to your requirements.
阅读全文
相关推荐





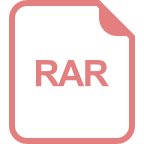
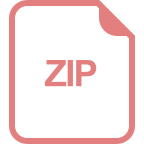
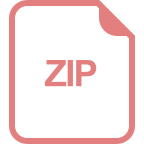
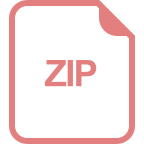






