那用这种方法反余弦函数怎么实现
时间: 2024-03-22 08:40:11 浏览: 23
在C语言中,反余弦函数可以使用math.h头文件中的acos函数来实现。acos函数的原型为:
```C
double acos(double x);
```
其中x为要求反余弦函数的值,函数返回值为x的反余弦值,单位为弧度。需要注意的是,参数x的取值范围必须在[-1,1]之间,否则函数会返回NaN。
例如,如果要求反余弦函数的值为0.5,可以使用以下代码:
```C
#include <math.h>
#include <stdio.h>
int main()
{
double x = 0.5;
double result = acos(x);
printf("acos(%f) = %f radians\n", x, result);
return 0;
}
```
输出:
```
acos(0.500000) = 1.047198 radians
```
相关问题
用这种方法求反余弦函数
求解反余弦函数可以使用类似的方法,同样可以使用牛顿迭代法来逼近反余弦函数的值。先来看一下反余弦函数的定义:
arccos(x) = y,其中 0 ≤ y ≤ π,且 cos(y) = x
我们可以对该式两侧同时求导,得到:
-arcsin'(cos(y)) = 1 / sqrt(1 - cos^2(y))
化简一下,得到:
sin(y) = sqrt(1 - cos^2(y))
代入原式中,得到:
arccos(x) = y = atan(sqrt(1 - x^2) / x)
其中 atan 表示求反正切函数。因此,我们可以用类似求反正弦函数的方式,使用牛顿迭代法逼近反余弦函数的值。
代码实现如下:
```c
#include<stdio.h>
#include<math.h>
double arccos(double x) {
double y = atan(sqrt(1 - x*x) / x); // 初始值
double eps = 1e-8; // 精度要求
int i = 0; // 迭代次数
while (1) {
double tmp = cos(y); // 临时变量
y = y - (tmp - x) / sin(y); // 牛顿迭代公式
if (fabs(cos(y) - tmp) < eps) // 满足精度要求
break;
i++;
if (i > 1000) // 迭代次数过多,可能陷入死循环,强制退出
break;
}
return y;
}
int main() {
double x = 0.5;
printf("arccos(%lf) = %lf\n", x, arccos(x));
return 0;
}
```
在主函数中,我们测试了求解反余弦函数在 x=0.5 时的值,并输出结果。
用这种方法求反正切函数
求解反正切函数可以使用类似的方法,同样可以使用牛顿迭代法来逼近反正切函数的值。先来看一下反正切函数的定义:
arctan(x) = y,其中 -π/2 < y < π/2,且 tan(y) = x
我们可以对该式两侧同时求导,得到:
arctan'(x) = 1 / (1 + x^2)
化简一下,得到:
tan(arctan(x))' = 1 / (1 + x^2)
代入原式中,得到:
arctan(x) = y = y - (tan(y) - x) / (1 + tan^2(y))
因此,我们可以用类似求反正弦函数和反余弦函数的方式,使用牛顿迭代法逼近反正切函数的值。
代码实现如下:
```c
#include<stdio.h>
#include<math.h>
double arctan(double x) {
double y = x; // 初始值
double eps = 1e-8; // 精度要求
int i = 0; // 迭代次数
while (1) {
double tmp = tan(y); // 临时变量
y = y - (tmp - x) / (1 + tmp*tmp); // 牛顿迭代公式
if (fabs(tan(y) - tmp) < eps) // 满足精度要求
break;
i++;
if (i > 1000) // 迭代次数过多,可能陷入死循环,强制退出
break;
}
return y;
}
int main() {
double x = 0.5;
printf("arctan(%lf) = %lf\n", x, arctan(x));
return 0;
}
```
在主函数中,我们测试了求解反正切函数在 x=0.5 时的值,并输出结果。
相关推荐
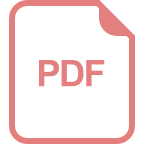
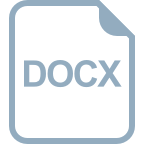
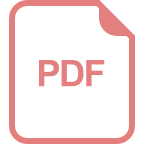










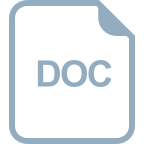
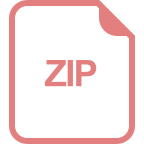
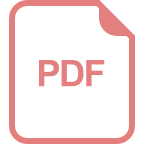