某小区物业为了更好的服务住户,为空巢老人和单亲家庭发放社区购物优惠证。空巢老人优惠证和单亲家庭可以享受购物打折优惠,具体优惠力度视家庭情况而定,空巢老人优惠证在8折的基础上,每多一个老人折扣减1到1.5之间不定(最多两个老人),单亲家庭优惠证在9折的基础上,每多一个孩子,折扣减0.5-1之间不定(最多5个孩子)。持证到物业处,输入家庭单人享受的减少折扣,可以查询优惠证的折扣力度。请依据题目完成下列题目: (1)设计优惠证抽象类Discount,包含家庭类型和享受优惠人数两个封装属性,为属性添加setter和getter方法;定义抽象方法返回优惠证可每次消费享受的折扣费用 public abstract double getDiscount(int peopleCount);其中peopleCount为单人享受的折扣减少折扣。(20分) 创建子类EmptyNestDiscount和SingleParentDiscount继承Discount类并实现getDiscount方法,依据优惠证类型和单人享受的减少折扣返回此优惠证的最终享受折扣(最终享受折扣=优惠证折扣基础 - 单人享受的减少折扣 * 享受优惠人数)(例如:空巢老人优惠证的单人享受减少折扣是1.2,家庭人数是2,那最终折扣为8-1.2*2=5.6折)。
时间: 2024-02-03 14:11:55 浏览: 19
```java
//定义抽象类Discount
public abstract class Discount {
private String familyType; //家庭类型
private int peopleCount; //享受优惠人数
public String getFamilyType() {
return familyType;
}
public void setFamilyType(String familyType) {
this.familyType = familyType;
}
public int getPeopleCount() {
return peopleCount;
}
public void setPeopleCount(int peopleCount) {
this.peopleCount = peopleCount;
}
public abstract double getDiscount(int peopleCount); //抽象方法,返回每次消费可享受的折扣费用
}
//创建子类EmptyNestDiscount
public class EmptyNestDiscount extends Discount {
@Override
public double getDiscount(int peopleCount) {
double baseDiscount = 0.8; //基础折扣为8折
if (getPeopleCount() == 1) { //1个老人家庭优惠折扣为8折
return baseDiscount - peopleCount;
} else if (getPeopleCount() > 1 && getPeopleCount() <= 2) { //多于1个老人家庭优惠折扣在8折基础上每增加一个老人折扣减少0.5-1之间不定
return baseDiscount - (1 + peopleCount * 0.5);
} else { //人数超过2个老人,不再享受折扣
return baseDiscount;
}
}
}
//创建子类SingleParentDiscount
public class SingleParentDiscount extends Discount {
@Override
public double getDiscount(int peopleCount) {
double baseDiscount = 0.9; //基础折扣为9折
if (getPeopleCount() == 1) { //1个孩子家庭优惠折扣为9折
return baseDiscount - peopleCount * 0.5;
} else if (getPeopleCount() > 1 && getPeopleCount() <= 5) { //多于1个孩子家庭优惠折扣在9折基础上每增加一个孩子折扣减少0.5-1之间不定
return baseDiscount - (peopleCount * 0.5);
} else { //孩子人数超过5个,不再享受折扣
return baseDiscount;
}
}
}
```
相关推荐
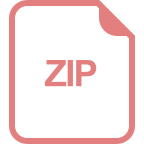
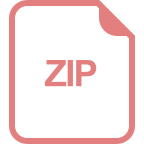
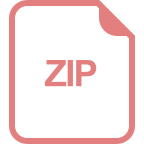















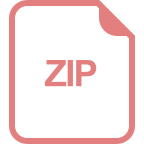