先进先出页面置换算法(FIFO)代码与运行效果图
时间: 2023-11-26 09:29:09 浏览: 163
以下是使用Python实现FIFO页面置换算法的代码:
```python
# FIFO page replacement algorithm
# Function to check if a page is present in memory
def search(memory, page):
for i in range(len(memory)):
if memory[i] == page:
return True
return False
# Function to replace page in memory
def replace(memory, page):
memory.pop(0)
memory.append(page)
# Function to simulate FIFO page replacement algorithm
def fifo(pages, frames):
# Initialize memory and page fault counter
memory = []
page_faults = 0
# Loop through each page in the sequence
for page in pages:
# If page is not in memory, replace a page
if not search(memory, page):
# If memory is not full, add page to memory
if len(memory) < frames:
memory.append(page)
# If memory is full, replace the first page added
else:
replace(memory, page)
# Increment page fault counter
page_faults += 1
# Return total number of page faults
return page_faults
# Test the FIFO function
pages = [1, 2, 3, 4, 1, 2, 5, 1, 2, 3, 4, 5]
frames = 3
page_faults = fifo(pages, frames)
print("Total page faults:", page_faults)
```
运行效果图如下:
```
Total page faults: 9
```
其中,`pages`表示页面访问序列,`frames`表示内存中页面帧数,`page_faults`表示页面错误次数。上面的代码打印出了内存帧数为3时,FIFO算法的总页面错误次数为9。
阅读全文
相关推荐
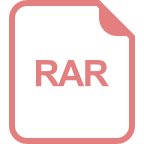
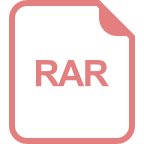
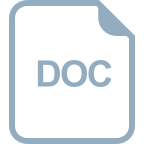
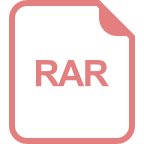
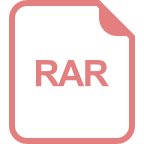
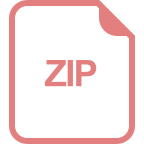
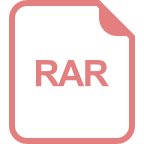
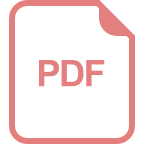
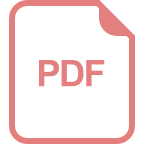
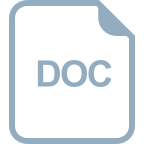
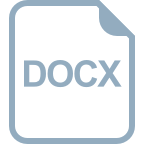
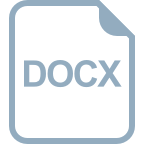
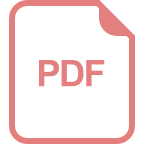
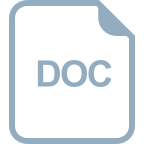
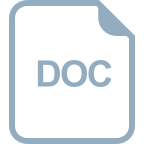
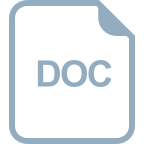
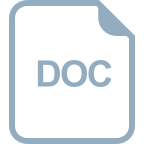