import subprocess # 原始图像路径 input_image = 'example.jpg' # 压缩后图像路径 output_image = 'example_compressed.jpg' # 压缩质量,取值范围0-100,值越高图像质量越好,文件大小也越大 quality = 80 # 使用cwebp命令行工具进行压缩 subprocess.run(['cwebp', input_image, '-q', str(quality), '-o', output_image]) # 计算压缩率 original_size = os.path.getsize(input_image) compressed_size = os.path.getsize(output_image) compression_ratio = original_size / compressed_size print('Compression ratio:', compression_ratio)
时间: 2024-02-22 14:00:52 浏览: 116
这段代码是使用`cwebp`命令行工具进行图像压缩,并计算压缩率的代码。如果你想要运行这个代码,需要将`example.jpg`替换为你要压缩的彩色图像文件路径,并将`example_compressed.jpg`替换为压缩后的图像文件路径。
另外,这段代码中使用了`os.path.getsize`函数来获取文件大小,需要先导入`os`模块,如下所示:
```python
import os
```
如果你在Windows上使用JupyterLab,需要先安装`webp`编码器,并将`cwebp`命令行工具添加到系统路径中。
相关问题
import tkinter as tkfrom tkinter import filedialogimport subprocessclass GUI(tk.Frame): def __init__(self, master=None): super().__init__(master) self.master = master self.master.title("软件界面") self.pack() self.path_label = tk.Label(self, text="输入路径:") self.path_label.pack() self.path_entry = tk.Entry(self) self.path_entry.pack() self.path_name_label = tk.Label(self, text="输入路径名:") self.path_name_label.pack() self.path_name_entry = tk.Entry(self) self.path_name_entry.pack() self.run_button = tk.Button(self, text="运行", command=self.run_program) self.run_button.pack() self.output_label = tk.Label(self, text="输出:") self.output_label.pack() self.output_text = tk.Text(self) self.output_text.pack() def run_program(self): path = self.path_entry.get() path_name = self.path_name_entry.get() cmd = ['python', 'yourprogram.py', path, path_name] result = subprocess.run(cmd, capture_output=True) self.output_text.insert(tk.END, result.stdout.decode())if __name__ == "__main__": root = tk.Tk() app = GUI(master=root) app.mainloop() 和import sysif __name__ == "__main__": # 获取从命令行传递过来的参数 path = sys.argv[1] path_name = sys.argv[2] # 在这里写你的程序逻辑 # ...这2个代码怎么关联不上
这两段代码是用来实现从界面输入路径和路径名,然后调用命令行运行Python程序的。具体来说,第一段代码是使用tkinter库创建了一个GUI界面,包括两个输入框、一个运行按钮和一个输出框。当用户点击运行按钮时,会获取输入框中的路径和路径名,并将其作为参数调用subprocess库运行Python程序。
而第二段代码则是一个独立的Python程序,它使用sys库获取命令行传递的参数,然后根据路径和路径名执行相应的程序逻辑。这段代码需要在第一段代码中调用,使用subprocess库运行该Python程序,并传递路径和路径名作为参数。具体来说,第一段代码的cmd变量中的'yourprogram.py'应该替换为第二段代码的Python程序文件名。
def mac_code_confirm(): import subprocess # 定义 PowerShell 命令 ps_command = r'Get-WmiObject Win32_ComputerSystemProduct | Select-Object -ExpandProperty UUID' # 执行 PowerShell 命令并获取输出 result = subprocess.run(['powershell', '-Command', ps_command], capture_output=True, text=True) # 检查 PowerShell 命令是否执行成功 if result.returncode == 0: machine_code = result.stdout.strip() expected_machine_code = "9E19AA0D-772D-C93B-3544-50EBF6592F65" if machine_code != expected_machine_code: print("未授权") import subprocess exit()优化这段代码
这段代码的作用是获取计算机的唯一标识符,并与预期的标识符进行比较,如果不匹配则输出未授权并退出程序。
可以对代码进行如下优化:
1. 使用 f-string 格式化字符串,可以使代码更加简洁、易读。
2. 使用 subprocess 的 check_output 方法,可以在命令执行失败时抛出异常。
3. 退出程序可以直接使用 sys 模块的 exit 函数,不需要使用 subprocess 调用 exit 命令。
优化后的代码如下所示:
```
import subprocess
import sys
def mac_code_confirm():
# 定义 PowerShell 命令
ps_command = r'Get-WmiObject Win32_ComputerSystemProduct | Select-Object -ExpandProperty UUID'
# 执行 PowerShell 命令并获取输出
try:
machine_code = subprocess.check_output(['powershell', '-Command', ps_command], text=True).strip()
except subprocess.CalledProcessError:
print("获取计算机唯一标识符失败")
sys.exit(1)
expected_machine_code = "9E19AA0D-772D-C93B-3544-50EBF6592F65"
if machine_code != expected_machine_code:
print("未授权")
sys.exit(1)
```
这样可以使代码更加简洁、易读,并且在错误处理上也更加健壮。
阅读全文
相关推荐
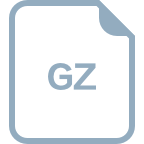
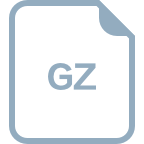
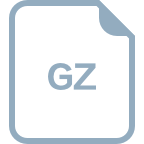













