C语言题目2 奇偶数分离 1. 问题描述 编写程序,产生100个0-200之间的随机数,在屏幕上显示出来,并将其中的奇数存储在odd.txt文件中,将偶数存储在even.txt文件中。 2. 代码(要求有注释)
时间: 2024-03-25 17:37:06 浏览: 17
```
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
int num, i;
FILE *odd_file, *even_file; // 定义两个文件指针
// 随机数种子
srand((unsigned) time(NULL));
// 打开两个文件
odd_file = fopen("odd.txt", "w");
even_file = fopen("even.txt", "w");
// 产生100个随机数并分离奇偶数
for (i = 0; i < 100; i++) {
num = rand() % 201; // 产生0-200之间的随机数
if (num % 2 == 0) {
fprintf(even_file, "%d ", num); // 写入偶数文件
} else {
fprintf(odd_file, "%d ", num); // 写入奇数文件
}
printf("%d ", num); // 在屏幕上显示随机数
}
// 关闭文件
fclose(odd_file);
fclose(even_file);
return 0;
}
```
注释解析:
1. `srand((unsigned) time(NULL));`:根据当前时间初始化随机数种子,确保每次运行程序生成的随机数不同。
2. `odd_file = fopen("odd.txt", "w");`:以写入方式打开odd.txt文件,如果文件不存在则创建。
3. `even_file = fopen("even.txt", "w");`:以写入方式打开even.txt文件,如果文件不存在则创建。
4. `num = rand() % 201;`:生成0-200之间的随机数。
5. `if (num % 2 == 0) {...}`:判断num是否为偶数,如果是,则写入even.txt文件。
6. `else {...}`:否则,写入odd.txt文件。
7. `fclose(odd_file);`:关闭odd.txt文件。
8. `fclose(even_file);`:关闭even.txt文件。
相关推荐
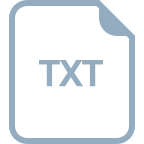














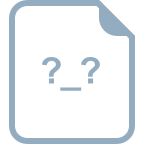