用Java写一个简单的推箱子游戏,注释要多多益善
时间: 2024-02-03 17:14:35 浏览: 116
好的,以下是一个简单的推箱子游戏的Java代码,注释已经尽可能详细,希望对你有帮助。
```java
import java.util.Scanner;
public class PushBoxGame {
public static void main(String[] args) {
// 初始化地图
char[][] map = {
{'#', '#', '#', '#', '#', '#', '#', '#', '#', '#'},
{'#', ' ', ' ', ' ', '#', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', '#', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', '#', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', '#', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'},
{'#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'},
{'#', '#', '#', '#', '#', '#', '#', '#', '#', '#'}
};
// 初始化箱子和目标位置
int boxRow = 4, boxCol = 4, targetRow = 2, targetCol = 8;
// 循环读取用户输入的方向,并更新地图、箱子、目标位置等信息
Scanner scanner = new Scanner(System.in);
while (true) {
// 打印地图
for (int i = 0; i < map.length; i++) {
for (int j = 0; j < map[i].length; j++) {
if (i == boxRow && j == boxCol) {
System.out.print('O'); // 箱子
} else if (i == targetRow && j == targetCol) {
System.out.print('X'); // 目标位置
} else {
System.out.print(map[i][j]);
}
}
System.out.println();
}
// 判断是否完成游戏
if (boxRow == targetRow && boxCol == targetCol) {
System.out.println("Congratulations! You win!");
break;
}
// 读取用户输入的方向
System.out.print("Please enter the direction (w/a/s/d): ");
String direction = scanner.nextLine();
// 根据方向更新箱子和目标位置等信息
int newRow = boxRow, newCol = boxCol; // 新的箱子位置
switch (direction) {
case "w":
newRow--;
break;
case "a":
newCol--;
break;
case "s":
newRow++;
break;
case "d":
newCol++;
break;
default:
System.out.println("Invalid direction!");
continue;
}
if (map[newRow][newCol] == ' ') { // 新的位置是空格,可以移动
map[boxRow][boxCol] = ' '; // 原来的位置变成空格
map[newRow][newCol] = 'O'; // 新的位置放置箱子
boxRow = newRow;
boxCol = newCol;
} else if (map[newRow][newCol] == 'X') { // 新的位置是目标位置,可以移动并完成游戏
map[boxRow][boxCol] = ' ';
map[newRow][newCol] = 'O';
boxRow = newRow;
boxCol = newCol;
} else { // 新的位置是障碍物,不能移动
System.out.println("Cannot move in this direction!");
}
}
}
}
```
阅读全文
相关推荐
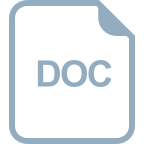
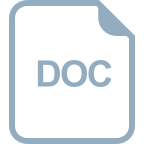
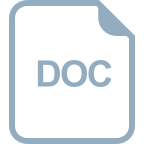
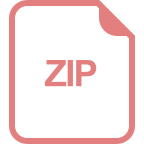
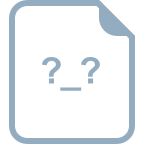
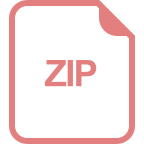
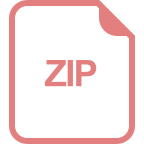
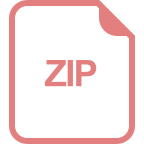
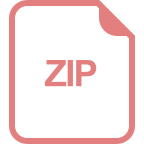
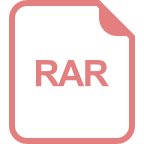
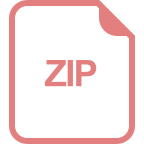
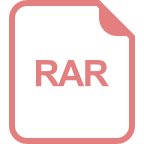
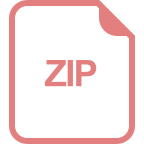
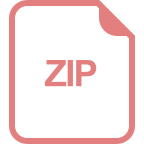
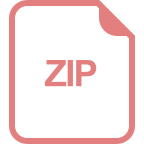
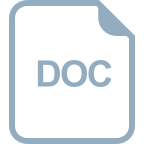
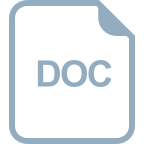
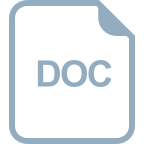
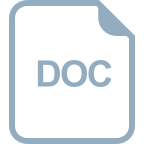