用python编写某品牌手机图片爬虫和评论数据分析,并进行数据获取:采集京东某品牌手机评论数据,数据预处理,可视化分析,图形用户界面,数据存取:使用txt/csv/excel等
时间: 2024-04-05 07:34:32 浏览: 36
好的,这是一个比较复杂的项目,需要分为多个步骤来完成。下面是一个简要的流程:
1. 确定爬取的手机品牌,使用 Python 的 requests 库和 BeautifulSoup 库爬取京东手机评论数据。可以使用以下代码:
```python
import requests
from bs4 import BeautifulSoup
url = "https://sclub.jd.com/comment/productPageComments.action?productId=123456&score=0&sortType=5&page=0&pageSize=10"
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, "html.parser")
```
其中,将上面的 url 中的 productId 替换为要爬取的手机品牌的 ID。
2. 解析评论数据,提取有用的信息。可以使用以下代码:
```python
comments = soup.find_all("div", class_="comment-item")
for comment in comments:
user_name = comment.find("div", class_="user-info").find("a").text.strip()
content = comment.find("div", class_="comment-content").find("p").text.strip()
score = comment.find("div", class_="comment-star").find("i")["class"][1][-1]
time = comment.find("div", class_="comment-op").find_all("span")[1].text.strip()
```
其中,user_name 表示用户名,content 表示评论内容,score 表示评分,time 表示评论时间。
3. 数据预处理,例如去除停用词、分词等。可以使用 jieba 库进行中文分词,使用以下代码:
```python
import jieba
jieba.set_dictionary("dict.txt.big")
comment_list = []
for comment in comments:
content = comment.find("div", class_="comment-content").find("p").text.strip()
seg_list = jieba.cut(content, cut_all=False)
comment_list.append(" ".join(seg_list))
```
其中,dict.txt.big 是 jieba 带的中文分词词典。
4. 进行数据分析,例如情感分析、词频统计等。可以使用情感分析库 TextBlob 进行情感分析,使用以下代码:
```python
from textblob import TextBlob
positive_count = 0
negative_count = 0
neutral_count = 0
for comment in comment_list:
blob = TextBlob(comment)
if blob.sentiment.polarity > 0:
positive_count += 1
elif blob.sentiment.polarity < 0:
negative_count += 1
else:
neutral_count += 1
```
其中,positive_count 表示积极评论数量,negative_count 表示消极评论数量,neutral_count 表示中性评论数量。
5. 进行可视化分析,例如绘制词云图、条形图等。可以使用词云库 wordcloud 和绘图库 matplotlib 进行可视化分析,使用以下代码:
```python
from wordcloud import WordCloud
import matplotlib.pyplot as plt
comment_text = " ".join(comment_list)
wordcloud = WordCloud(background_color="white", width=800, height=400, max_words=100, font_path="msyh.ttc").generate(comment_text)
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis("off")
plt.show()
```
其中,msyh.ttc 是字体文件,用于绘制中文词云。
6. 使用 PyQT5 库构建图形用户界面,方便用户输入爬取的手机品牌和保存的文件名等信息,使用以下代码:
```python
from PyQt5 import QtWidgets, QtGui, QtCore
class MainWindow(QtWidgets.QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle("手机评论数据分析")
self.resize(400, 300)
self.brand_label = QtWidgets.QLabel("手机品牌")
self.brand_edit = QtWidgets.QLineEdit()
self.file_label = QtWidgets.QLabel("保存文件名")
self.file_edit = QtWidgets.QLineEdit()
self.start_button = QtWidgets.QPushButton("开始爬取")
self.start_button.clicked.connect(self.start_crawl)
layout = QtWidgets.QVBoxLayout()
layout.addWidget(self.brand_label)
layout.addWidget(self.brand_edit)
layout.addWidget(self.file_label)
layout.addWidget(self.file_edit)
layout.addWidget(self.start_button)
self.setLayout(layout)
def start_crawl(self):
brand = self.brand_edit.text()
file_name = self.file_edit.text()
# 爬取和分析数据的代码
# ...
if __name__ == "__main__":
app = QtWidgets.QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
其中,MainWindow 类继承自 QWidget 类,实现了图形用户界面的各个部分,start_crawl 方法用于处理用户输入的信息并调用爬取和分析数据的代码。
7. 使用 pandas 库将数据存储到 txt/csv/excel 等文件中,使用以下代码:
```python
import pandas as pd
df = pd.DataFrame({"user_name": user_names, "content": comment_list, "score": scores, "time": times})
df.to_csv(file_name + ".csv", index=False)
```
其中,user_names、comment_list、scores 和 times 分别表示用户名、评论内容、评分和评论时间,df 表示将这些数据存储到一个 DataFrame 中,to_csv 方法将 DataFrame 存储到 csv 文件中。可以将 to_csv 替换为 to_excel、to_json 等方法,根据需要将数据存储到不同格式的文件中。
以上是一个简要的流程,实际上还需要考虑一些细节问题,例如反爬虫、数据清洗等。同时,需要根据具体需求进行修改和优化。
相关推荐
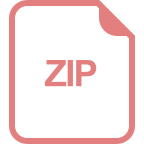
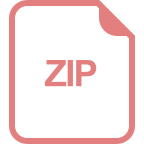
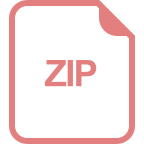

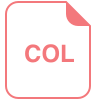
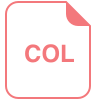
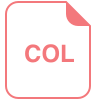










