windows下C语言使用openssl库生成rsa密钥对进行加解密并给出源代码
时间: 2023-12-28 17:03:36 浏览: 169
好的,以下是使用openssl库在Windows下用C语言生成RSA密钥对并进行加解密的源代码。需要注意的是,以下代码需要在包含openssl库的环境下编译运行。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <openssl/err.h>
#define KEY_LENGTH 2048
#define PUB_EXP 3
#define MESSAGE_SIZE 1024
int main()
{
RSA *keypair = RSA_generate_key(KEY_LENGTH, PUB_EXP, NULL, NULL);
unsigned char plaintext[MESSAGE_SIZE] = "This is a message to be encrypted";
unsigned char ciphertext[MESSAGE_SIZE];
unsigned char decryptedtext[MESSAGE_SIZE];
int ciphertext_len, decryptedtext_len;
// 加密过程
ciphertext_len = RSA_public_encrypt(strlen(plaintext)+1, plaintext, ciphertext, keypair, RSA_PKCS1_PADDING);
if(ciphertext_len == -1)
{
printf("加密失败: %s\n", ERR_error_string(ERR_get_error(), NULL));
exit(EXIT_FAILURE);
}
// 打印加密结果
printf("加密后的密文:\n");
for(int i = 0; i < ciphertext_len; i++)
{
printf("%02x ", ciphertext[i]);
}
printf("\n");
// 解密过程
decryptedtext_len = RSA_private_decrypt(ciphertext_len, ciphertext, decryptedtext, keypair, RSA_PKCS1_PADDING);
if(decryptedtext_len == -1)
{
printf("解密失败: %s\n", ERR_error_string(ERR_get_error(), NULL));
exit(EXIT_FAILURE);
}
// 打印解密结果
printf("解密后的明文:%s\n", decryptedtext);
RSA_free(keypair);
return 0;
}
```
以上代码使用openssl库生成了一个2048位的RSA密钥对,并使用公钥加密了一段文本,再使用私钥进行解密。您可以根据需要进行修改。
阅读全文
相关推荐
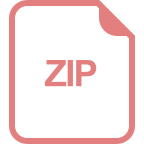

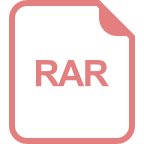
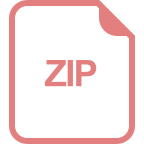
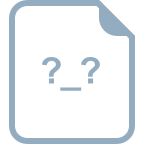
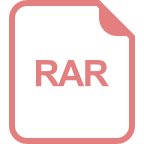
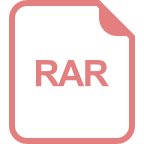
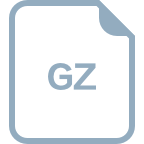
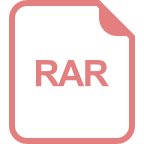
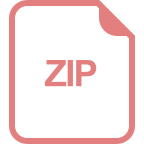
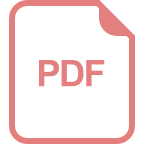
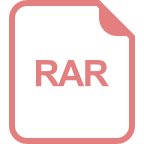
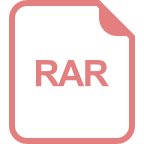
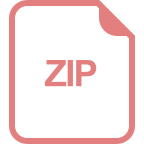
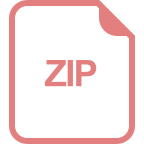
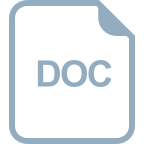
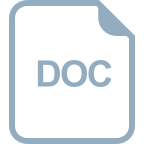