编写ado.net学生管理系统
时间: 2023-06-13 13:02:34 浏览: 199
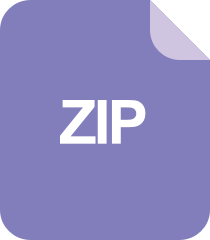
ASP.NET学生管理系统.zip
这里给出一个简单的ADO.NET学生管理系统示例,包含以下功能:
1. 添加学生信息
2. 修改学生信息
3. 删除学生信息
4. 查询学生信息
首先,需要建立数据库表,这里建立一个名为"Students"的表,包含以下字段:
- StudentID (int, primary key)
- Name (nvarchar(50))
- Age (int)
- Gender (nvarchar(10))
- Major (nvarchar(50))
接下来,创建一个Visual Studio项目,选择C#语言,类型选择“Windows 窗体应用程序”。在解决方案资源管理器中,右键单击项目,选择“添加 - 新建项”,选择“数据”类别,然后选择“数据集”,命名为“StudentDataSet”。
在“Data Sources”窗口中,选择“新建数据集”,然后将“Students”表添加到数据集中。此时可以在设计器中进行表格的设计和布局。
接下来,创建一个窗体,添加文本框和按钮,用于添加、修改、删除和查询学生信息。在窗体代码中,使用ADO.NET连接数据库并实现相应的增删改查操作。
以下是完整的代码示例:
```csharp
using System;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace StudentManagementSystem
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void btnAdd_Click(object sender, EventArgs e)
{
// 获取用户输入的学生信息
string name = txtName.Text;
int age = int.Parse(txtAge.Text);
string gender = txtGender.Text;
string major = txtMajor.Text;
// 连接数据库
SqlConnection conn = new SqlConnection("Data Source=(local);Initial Catalog=StudentDB;Integrated Security=True");
conn.Open();
// 执行插入命令
SqlCommand cmd = new SqlCommand("INSERT INTO Students (Name, Age, Gender, Major) VALUES (@Name, @Age, @Gender, @Major)", conn);
cmd.Parameters.AddWithValue("@Name", name);
cmd.Parameters.AddWithValue("@Age", age);
cmd.Parameters.AddWithValue("@Gender", gender);
cmd.Parameters.AddWithValue("@Major", major);
cmd.ExecuteNonQuery();
// 关闭连接
conn.Close();
// 清空文本框
txtName.Text = "";
txtAge.Text = "";
txtGender.Text = "";
txtMajor.Text = "";
// 刷新数据
RefreshData();
}
private void btnUpdate_Click(object sender, EventArgs e)
{
// 获取用户输入的学生信息
int id = int.Parse(txtID.Text);
string name = txtName.Text;
int age = int.Parse(txtAge.Text);
string gender = txtGender.Text;
string major = txtMajor.Text;
// 连接数据库
SqlConnection conn = new SqlConnection("Data Source=(local);Initial Catalog=StudentDB;Integrated Security=True");
conn.Open();
// 执行更新命令
SqlCommand cmd = new SqlCommand("UPDATE Students SET Name=@Name, Age=@Age, Gender=@Gender, Major=@Major WHERE StudentID=@ID", conn);
cmd.Parameters.AddWithValue("@ID", id);
cmd.Parameters.AddWithValue("@Name", name);
cmd.Parameters.AddWithValue("@Age", age);
cmd.Parameters.AddWithValue("@Gender", gender);
cmd.Parameters.AddWithValue("@Major", major);
cmd.ExecuteNonQuery();
// 关闭连接
conn.Close();
// 清空文本框
txtID.Text = "";
txtName.Text = "";
txtAge.Text = "";
txtGender.Text = "";
txtMajor.Text = "";
// 刷新数据
RefreshData();
}
private void btnDelete_Click(object sender, EventArgs e)
{
// 获取用户输入的学生ID
int id = int.Parse(txtID.Text);
// 连接数据库
SqlConnection conn = new SqlConnection("Data Source=(local);Initial Catalog=StudentDB;Integrated Security=True");
conn.Open();
// 执行删除命令
SqlCommand cmd = new SqlCommand("DELETE FROM Students WHERE StudentID=@ID", conn);
cmd.Parameters.AddWithValue("@ID", id);
cmd.ExecuteNonQuery();
// 关闭连接
conn.Close();
// 清空文本框
txtID.Text = "";
txtName.Text = "";
txtAge.Text = "";
txtGender.Text = "";
txtMajor.Text = "";
// 刷新数据
RefreshData();
}
private void btnSearch_Click(object sender, EventArgs e)
{
// 获取用户输入的学生ID
int id = int.Parse(txtID.Text);
// 连接数据库
SqlConnection conn = new SqlConnection("Data Source=(local);Initial Catalog=StudentDB;Integrated Security=True");
conn.Open();
// 执行查询命令
SqlCommand cmd = new SqlCommand("SELECT * FROM Students WHERE StudentID=@ID", conn);
cmd.Parameters.AddWithValue("@ID", id);
SqlDataReader reader = cmd.ExecuteReader();
// 显示查询结果
if (reader.Read())
{
txtName.Text = reader["Name"].ToString();
txtAge.Text = reader["Age"].ToString();
txtGender.Text = reader["Gender"].ToString();
txtMajor.Text = reader["Major"].ToString();
}
else
{
MessageBox.Show("未找到该学生信息!");
}
// 关闭连接
conn.Close();
}
private void RefreshData()
{
// 连接数据库
SqlConnection conn = new SqlConnection("Data Source=(local);Initial Catalog=StudentDB;Integrated Security=True");
conn.Open();
// 执行查询命令
SqlCommand cmd = new SqlCommand("SELECT * FROM Students", conn);
SqlDataReader reader = cmd.ExecuteReader();
// 显示查询结果
dgvStudents.Rows.Clear();
while (reader.Read())
{
dgvStudents.Rows.Add(reader["StudentID"], reader["Name"], reader["Age"], reader["Gender"], reader["Major"]);
}
// 关闭连接
conn.Close();
}
}
}
```
以上代码实现了一个简单的ADO.NET学生管理系统。注意,这只是一个示例,实际应用中需要考虑更多的异常处理和安全性问题。
阅读全文
相关推荐
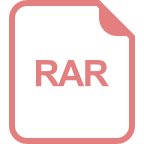















