C语言多线程程序
时间: 2023-07-07 12:11:41 浏览: 58
在C语言中,可以使用多线程来实现在一个程序中同时执行多个任务的目的。C语言中实现多线程需要使用线程库,常见的线程库有POSIX线程库和Windows线程库。下面以POSIX线程库为例,介绍如何在C语言中编写多线程程序。
首先需要包含头文件pthread.h,然后使用pthread_create函数创建一个新线程,函数的语法如下:
```
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine)(void *), void *arg);
```
其中,thread是指向线程标识符的指针,attr是指向线程属性的指针,start_routine是指向线程函数的指针,arg是传递给线程函数的参数。
线程函数的形式如下:
```
void *thread_func(void *arg);
```
其中,arg是传递给线程函数的参数。
下面是一个简单的多线程程序示例:
```
#include <stdio.h>
#include <pthread.h>
void *thread_func(void *arg)
{
int i;
for (i = 0; i < 10; i++) {
printf("Thread %d: %d\n", *((int *)arg), i);
}
return NULL;
}
int main()
{
pthread_t thread1, thread2;
int arg1 = 1, arg2 = 2;
pthread_create(&thread1, NULL, thread_func, &arg1);
pthread_create(&thread2, NULL, thread_func, &arg2);
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
return 0;
}
```
上述代码创建了两个线程,分别执行thread_func函数,并传递不同的参数(1和2)给每个线程。在thread_func函数中,使用for循环输出线程号和计数器的值。在main函数中,调用pthread_join函数等待线程执行完毕,并回收线程资源。
需要注意的是,在多线程程序中,多个线程共享进程的内存空间,因此需要注意线程之间的同步和互斥问题,以避免线程间的竞争和冲突。
相关推荐
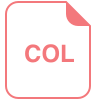
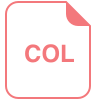
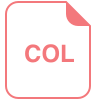
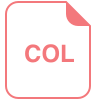
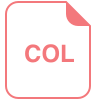









