用matlab编程实现彩色图片中三角形的检测
时间: 2024-04-22 17:22:49 浏览: 14
实现彩色图片中三角形的检测,可以采用以下步骤:
1. 读入彩色图片并进行灰度化处理。
```matlab
img = imread('color_image.jpg');
gray_img = rgb2gray(img);
```
2. 对灰度图像进行边缘检测,可以使用Canny算子或Sobel算子等。
```matlab
edge_img = edge(gray_img, 'Canny');
```
3. 对边缘图像进行霍夫变换,检测直线。
```matlab
[H, T, R] = hough(edge_img);
P = houghpeaks(H, 50);
lines = houghlines(edge_img, T, R, P);
```
4. 根据直线的交点,计算出所有可能的三角形,并进行筛选。
```matlab
for i = 1:length(lines)-2
for j = i+1:length(lines)-1
for k = j+1:length(lines)
x1 = lines(i).point1(1);
y1 = lines(i).point1(2);
x2 = lines(i).point2(1);
y2 = lines(i).point2(2);
x3 = lines(j).point1(1);
y3 = lines(j).point1(2);
x4 = lines(j).point2(1);
y4 = lines(j).point2(2);
x5 = lines(k).point1(1);
y5 = lines(k).point1(2);
x6 = lines(k).point2(1);
y6 = lines(k).point2(2);
if abs(atan2(y2-y1, x2-x1) - atan2(y4-y3, x4-x3)) < 0.1 && abs(atan2(y2-y1, x2-x1) - atan2(y6-y5, x6-x5)) < 0.1
if abs(sqrt((x1-x3)^2 + (y1-y3)^2) - sqrt((x2-x4)^2 + (y2-y4)^2)) < 5 && abs(sqrt((x1-x5)^2 + (y1-y5)^2) - sqrt((x2-x6)^2 + (y2-y6)^2)) < 5
triangle = [x1, y1, x2, y2, x3, y3, x4, y4, x5, y5, x6, y6];
triangles = [triangles; triangle];
end
end
end
end
end
```
其中,筛选的条件是:三条直线的夹角小于0.1弧度,且三边的长度差异小于5个像素。
5. 在原图上绘制出所有检测到的三角形。
```matlab
figure, imshow(img), hold on
for i = 1:size(triangles, 1)
x = triangles(i, [1,3,5,1]);
y = triangles(i, [2,4,6,2]);
plot(x, y, 'LineWidth', 2, 'Color', 'r');
end
hold off
```
绘制出来的三角形可能不够精确,需要根据具体情况进行调整。
完整代码如下:
```matlab
img = imread('color_image.jpg');
gray_img = rgb2gray(img);
edge_img = edge(gray_img, 'Canny');
[H, T, R] = hough(edge_img);
P = houghpeaks(H, 50);
lines = houghlines(edge_img, T, R, P);
triangles = [];
for i = 1:length(lines)-2
for j = i+1:length(lines)-1
for k = j+1:length(lines)
x1 = lines(i).point1(1);
y1 = lines(i).point1(2);
x2 = lines(i).point2(1);
y2 = lines(i).point2(2);
x3 = lines(j).point1(1);
y3 = lines(j).point1(2);
x4 = lines(j).point2(1);
y4 = lines(j).point2(2);
x5 = lines(k).point1(1);
y5 = lines(k).point1(2);
x6 = lines(k).point2(1);
y6 = lines(k).point2(2);
if abs(atan2(y2-y1, x2-x1) - atan2(y4-y3, x4-x3)) < 0.1 && abs(atan2(y2-y1, x2-x1) - atan2(y6-y5, x6-x5)) < 0.1
if abs(sqrt((x1-x3)^2 + (y1-y3)^2) - sqrt((x2-x4)^2 + (y2-y4)^2)) < 5 && abs(sqrt((x1-x5)^2 + (y1-y5)^2) - sqrt((x2-x6)^2 + (y2-y6)^2)) < 5
triangle = [x1, y1, x2, y2, x3, y3, x4, y4, x5, y5, x6, y6];
triangles = [triangles; triangle];
end
end
end
end
end
figure, imshow(img), hold on
for i = 1:size(triangles, 1)
x = triangles(i, [1,3,5,1]);
y = triangles(i, [2,4,6,2]);
plot(x, y, 'LineWidth', 2, 'Color', 'r');
end
hold off
```
相关推荐
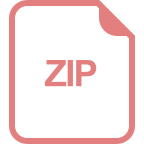
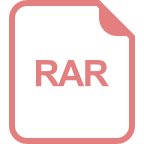














