python字符串中查找有几个子字符串
时间: 2023-09-12 18:10:34 浏览: 189
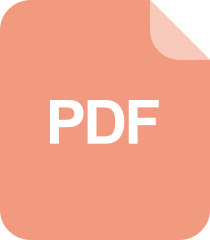
python实现在字符串中查找子字符串的方法
可以使用count方法统计一个字符串中子字符串出现的次数,示例如下:
```python
s = 'hello world'
sub_str = 'l'
count = s.count(sub_str)
print(count) # 输出 3
```
如果要统计多个子字符串出现的总次数,可以使用循环遍历每个子字符串,然后累加计数,示例如下:
```python
s = 'hello world'
sub_str_list = ['l', 'o']
count = 0
for sub_str in sub_str_list:
count += s.count(sub_str)
print(count) # 输出 4
```
这里统计了字符串中'l'和'o'出现的总次数。
阅读全文
相关推荐
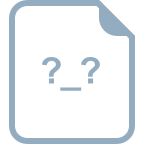
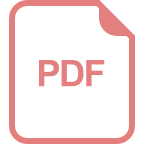
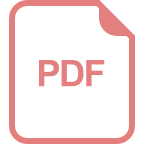
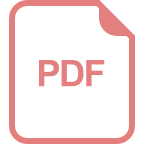
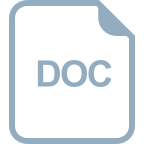
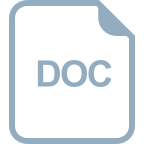
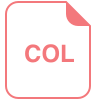
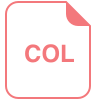
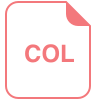







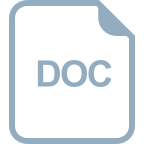
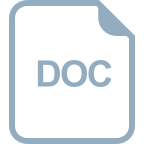