async function InProcessCount() { const list = await store.dispatch('ESourcingModule/GetMyRequest', searchView.value) // eslint-disable-next-line @typescript-eslint/no-unsafe-call event.InProcessCount = list.filter((o: { ViewType: string }) => o.ViewType === 'InClarification' || o.ViewType === 'SupplementEventDetails').length } function CheckAdmin(routeritem: RouteRecordRaw) { document.title = 'RMS E-Sourcing' let temp = event.UsersInfo if (routeritem !== undefined && routeritem.meta !== undefined && routeritem.meta?.title !== undefined && routeritem.meta?.title === 'My Request') { // eslint-disable-next-line @typescript-eslint/restrict-plus-operands routeritem.meta.title = routeritem.meta?.title + '(' + event.InProcessCount + ')' } const routeRoles = routeritem.meta?.roles as string[] !== undefined ? routeritem.meta?.roles as string[] : [] if (temp === undefined) { return false } for (let i = 0; i < temp.ROLES.length; i++) { if (routeRoles.indexOf(temp.ROLES[i]) > -1 && routeritem.meta?.isSub !== 'true') { // 路由包含当前用户权限,直接返回 return true } } return false } 优化代码
时间: 2024-02-14 07:04:02 浏览: 25
你的代码可以做一些优化,这样可以提高代码的可读性和性能。这里提供一份优化后的代码:
```
async function getInProcessCount() {
const list = await store.dispatch('ESourcingModule/GetMyRequest', searchView.value)
return list.filter((o: { ViewType: string }) => o.ViewType === 'InClarification' || o.ViewType === 'SupplementEventDetails').length
}
function checkAdmin(routeritem: RouteRecordRaw) {
document.title = 'RMS E-Sourcing'
// 获取 InProcessCount 数据
let inProcessCount = 0
if (routeritem?.meta?.title === 'My Request') {
getInProcessCount().then(count => inProcessCount = count)
}
// 检查用户权限
const userRoles = event.UsersInfo?.ROLES
const routeRoles = routeritem?.meta?.roles || []
if (!userRoles) {
return false
}
return userRoles.some(role => routeRoles.includes(role) && routeritem?.meta?.isSub !== 'true')
}
// 用法示例
const routerItem = { meta: { title: 'My Request', roles: ['admin'], isSub: false } }
checkAdmin(routerItem).then(isAdmin => {
if (isAdmin) {
routerItem.meta.title = `${routerItem.meta.title} (${inProcessCount})`
}
})
```
这里的优化主要有以下几点:
1. 把获取 InProcessCount 数据的操作封装成了一个独立的函数 `getInProcessCount()`,方便代码复用和维护。
2. 使用 Promise 和 async/await 异步获取数据,避免了数据无法同步的问题。
3. 使用了可选链运算符 `?.` 和空值合并运算符 `||`,简化了代码判断逻辑,提高了代码的可读性。
4. 在检查用户权限时,使用了 `Array.prototype.some()` 方法和 `Array.prototype.includes()` 方法,简化了代码逻辑,提高了性能。
另外,建议你在代码中使用一致的命名风格,比如使用小驼峰式命名方法名和变量名,使用大驼峰式命名类型名和类名,这样可以提高代码的可读性。
相关推荐
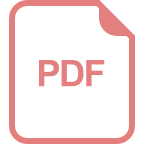
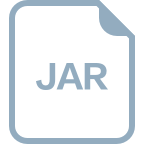
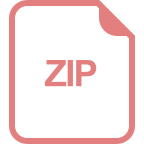














