3、由多个类组成的Java程序的编写与运行; (1)在FirstProgram.java中添加以下代码; public class Another{ public void say(){ System.out.println("Another one."); } } (2)使用javac命令编译程序,并根据出现的提示信息修改程序直至程序编译正确为止; (3)使用java命令运行程序,并根据出现的提示信息修改程序直至程序运行正确为止。 (4)请修改程序,使用Another类,使得“Another one”能够正常输出。
时间: 2023-06-26 18:10:10 浏览: 40
修改后的程序如下:
```java
public class FirstProgram {
public static void main(String[] args) {
Another another = new Another();
another.say();
}
}
class Another {
public void say() {
System.out.println("Another one.");
}
}
```
编译程序的命令为:`javac FirstProgram.java`
运行程序的命令为:`java FirstProgram`
运行正确后,输出结果为:`Another one.`
相关问题
实验任务1、2、3、4、5的JAVA运行代码
由于引用内容较多,无法在此直接提供完整的JAVA运行代码,建议参考引用中提供的实验报告模板及示例代码进行编写。以下是对每个实验任务的简要描述和相关参考代码:
实验任务1:设计一个GUI应用程序,窗口标题为“First GUI Program”,窗口大小为(500,300),窗口背景色为红色,居中显示。参考代码如下:
```java
import javax.swing.*;
public class FirstGUIProgram extends JFrame {
public FirstGUIProgram() {
setSize(500, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setTitle("First GUI Program");
getContentPane().setBackground(Color.RED);
setLocationRelativeTo(null);
}
public static void main(String[] args) {
FirstGUIProgram frame = new FirstGUIProgram();
frame.setVisible(true);
}
}
```
实验任务2:在实验任务1的基础上,响应按钮事件。当用户点击“green”按钮时,修改背景色为绿色。参考代码如下:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class FirstGUIProgram extends JFrame implements ActionListener {
private JButton btnGreen;
public FirstGUIProgram() {
setSize(500, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setTitle("First GUI Program");
getContentPane().setBackground(Color.RED);
setLocationRelativeTo(null);
JPanel panel = new JPanel();
getContentPane().add(panel, BorderLayout.SOUTH);
btnGreen = new JButton("green");
btnGreen.addActionListener(this);
panel.add(btnGreen);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btnGreen) {
getContentPane().setBackground(Color.GREEN);
}
}
public static void main(String[] args) {
FirstGUIProgram frame = new FirstGUIProgram();
frame.setVisible(true);
}
}
```
实验任务3:在实验任务2的基础上,添加一个按钮,当用户点击“reset”按钮时,将背景色还原为红色。参考代码如下:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class FirstGUIProgram extends JFrame implements ActionListener {
private JButton btnGreen;
private JButton btnReset;
public FirstGUIProgram() {
setSize(500, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setTitle("First GUI Program");
getContentPane().setBackground(Color.RED);
setLocationRelativeTo(null);
JPanel panel = new JPanel();
getContentPane().add(panel, BorderLayout.SOUTH);
btnGreen = new JButton("green");
btnGreen.addActionListener(this);
panel.add(btnGreen);
btnReset = new JButton("reset");
btnReset.addActionListener(this);
panel.add(btnReset);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btnGreen) {
getContentPane().setBackground(Color.GREEN);
} else if (e.getSource() == btnReset) {
getContentPane().setBackground(Color.RED);
}
}
public static void main(String[] args) {
FirstGUIProgram frame = new FirstGUIProgram();
frame.setVisible(true);
}
}
```
实验任务4:在实验任务3的基础上,添加两个文本框和两个标签,分别用于输入长和宽,并显示面积和周长。参考代码如下:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class FirstGUIProgram extends JFrame implements ActionListener {
private JButton btnGreen;
private JButton btnReset;
private JLabel lblLength;
private JLabel lblWidth;
private JTextField txtLength;
private JTextField txtWidth;
private JLabel lblArea;
private JLabel lblPerimeter;
public FirstGUIProgram() {
setSize(500, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setTitle("First GUI Program");
getContentPane().setBackground(Color.RED);
setLocationRelativeTo(null);
JPanel panel = new JPanel();
getContentPane().add(panel, BorderLayout.SOUTH);
btnGreen = new JButton("green");
btnGreen.addActionListener(this);
panel.add(btnGreen);
btnReset = new JButton("reset");
btnReset.addActionListener(this);
panel.add(btnReset);
JPanel centerPanel = new JPanel(new GridLayout(3, 2));
getContentPane().add(centerPanel, BorderLayout.CENTER);
lblLength = new JLabel("Length:");
centerPanel.add(lblLength);
txtLength = new JTextField();
centerPanel.add(txtLength);
lblWidth = new JLabel("Width:");
centerPanel.add(lblWidth);
txtWidth = new JTextField();
centerPanel.add(txtWidth);
JLabel lblAreaTitle = new JLabel("Area:");
centerPanel.add(lblAreaTitle);
lblArea = new JLabel();
centerPanel.add(lblArea);
JLabel lblPerimeterTitle = new JLabel("Perimeter:");
centerPanel.add(lblPerimeterTitle);
lblPerimeter = new JLabel();
centerPanel.add(lblPerimeter);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btnGreen) {
getContentPane().setBackground(Color.GREEN);
} else if (e.getSource() == btnReset) {
getContentPane().setBackground(Color.RED);
}
if (txtLength.getText().isEmpty() || txtWidth.getText().isEmpty()) {
lblArea.setText("");
lblPerimeter.setText("");
return;
}
double length = Double.parseDouble(txtLength.getText());
double width = Double.parseDouble(txtWidth.getText());
Rectangle rectangle = new Rectangle(length, width);
lblArea.setText(Double.toString(rectangle.getArea()));
lblPerimeter.setText(Double.toString(rectangle.getPerimeter()));
}
public static void main(String[] args) {
FirstGUIProgram frame = new FirstGUIProgram();
frame.setVisible(true);
}
}
class Rectangle {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
public double getLength() {
return length;
}
public double getWidth() {
return width;
}
public double getArea() {
return length * width;
}
public double getPerimeter() {
return 2 * (length + width);
}
}
```
实验任务5:在实验任务4的基础上,添加一个按钮,当用户点击“red”按钮时,将背景色变为红色,并清空所有文本框和标签。参考代码如下:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class FirstGUIProgram extends JFrame implements ActionListener {
private JButton btnGreen;
private JButton btnReset;
private JButton btnRed;
private JLabel lblLength;
private JLabel lblWidth;
private JTextField txtLength;
private JTextField txtWidth;
private JLabel lblArea;
private JLabel lblPerimeter;
public FirstGUIProgram() {
setSize(500, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setTitle("First GUI Program");
getContentPane().setBackground(Color.RED);
setLocationRelativeTo(null);
JPanel panel = new JPanel();
getContentPane().add(panel, BorderLayout.SOUTH);
btnGreen = new JButton("green");
btnGreen.addActionListener(this);
panel.add(btnGreen);
btnReset = new JButton("reset");
btnReset.addActionListener(this);
panel.add(btnReset);
btnRed = new JButton("red");
btnRed.addActionListener(this);
panel.add(btnRed);
JPanel centerPanel = new JPanel(new GridLayout(3, 2));
getContentPane().add(centerPanel, BorderLayout.CENTER);
lblLength = new JLabel("Length:");
centerPanel.add(lblLength);
txtLength = new JTextField();
centerPanel.add(txtLength);
lblWidth = new JLabel("Width:");
centerPanel.add(lblWidth);
txtWidth = new JTextField();
centerPanel.add(txtWidth);
JLabel lblAreaTitle = new JLabel("Area:");
centerPanel.add(lblAreaTitle);
lblArea = new JLabel();
centerPanel.add(lblArea);
JLabel lblPerimeterTitle = new JLabel("Perimeter:");
centerPanel.add(lblPerimeterTitle);
lblPerimeter = new JLabel();
centerPanel.add(lblPerimeter);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btnGreen) {
getContentPane().setBackground(Color.GREEN);
} else if (e.getSource() == btnReset) {
getContentPane().setBackground(Color.RED);
txtLength.setText("");
txtWidth.setText("");
lblArea.setText("");
lblPerimeter.setText("");
} else if (e.getSource() == btnRed) {
getContentPane().setBackground(Color.RED);
txtLength.setText("");
txtWidth.setText("");
lblArea.setText("");
lblPerimeter.setText("");
}
if (txtLength.getText().isEmpty() || txtWidth.getText().isEmpty()) {
lblArea.setText("");
lblPerimeter.setText("");
return;
}
double length = Double.parseDouble(txtLength.getText());
double width = Double.parseDouble(txtWidth.getText());
Rectangle rectangle = new Rectangle(length, width);
lblArea.setText(Double.toString(rectangle.getArea()));
lblPerimeter.setText(Double.toString(rectangle.getPerimeter()));
}
public static void main(String[] args) {
FirstGUIProgram frame = new FirstGUIProgram();
frame.setVisible(true);
}
}
class Rectangle {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
public double getLength() {
return length;
}
public double getWidth() {
return width;
}
public double getArea() {
return length * width;
}
public double getPerimeter() {
return 2 * (length + width);
}
}
```
阅读全文
相关推荐
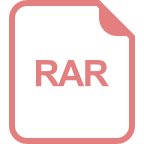
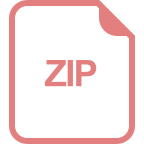
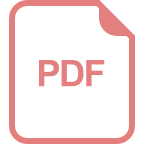
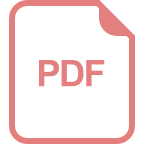
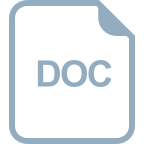
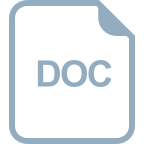
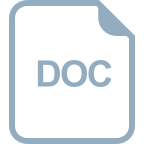
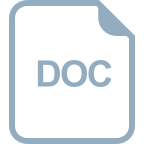
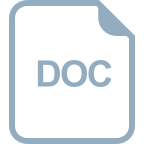
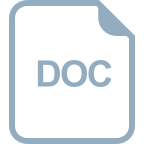
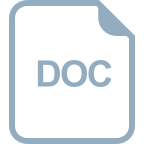
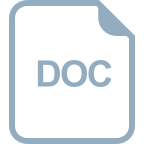
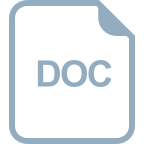
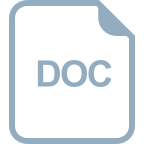
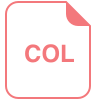
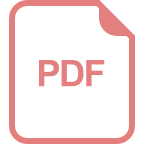