请帮我编写一个运用Easyx的扫雷游戏基于C语言
时间: 2023-06-07 18:11:34 浏览: 117
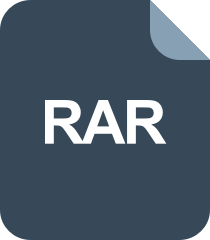
基于EasyX的C扫雷代码

好的,我可以为您提供以下代码示例:
```c
#include <graphics.h>
#include <conio.h>
#include <time.h>
#include <stdlib.h>
#define N 20 // 棋盘行列数
#define BLOCK_SIZE 30 // 每个方块大小
#define BOMB_CNT 50 // 炸弹数量
int map[N][N]; // 存储棋盘信息
int vis[N][N]; // 存储单元格开启状态
int bomb_x[BOMB_CNT], bomb_y[BOMB_CNT]; // 存储炸弹的位置
void init_map()
{
srand((unsigned)time(NULL));
for (int i = 0; i < BOMB_CNT; ++i) {
int x = rand() % N, y = rand() % N;
// 如果这个位置已有炸弹,则重新生成随机位置
while (map[x][y] == 9) {
x = rand() % N;
y = rand() % N;
}
map[x][y] = 9; // 在该位置放置炸弹
bomb_x[i] = x;
bomb_y[i] = y;
// 将该位置周围的单元格数字加1
for (int dx = -1; dx <= 1; ++dx) {
for (int dy = -1; dy <= 1; ++dy) {
int nx = x + dx, ny = y + dy;
if (nx < 0 || nx >= N || ny < 0 || ny >= N) continue; // 边界判断
if (map[nx][ny] != 9) map[nx][ny]++;
}
}
}
}
void draw_board()
{
setbkcolor(WHITE);
cleardevice();
for (int i = 0; i < N; ++i) {
for (int j = 0; j < N; ++j) {
if (vis[i][j] == 0) {
setfillcolor(LIGHTGRAY);
} else {
setfillcolor(WHITE);
}
int x = BLOCK_SIZE * j, y = BLOCK_SIZE * i;
fillrectangle(x, y, x + BLOCK_SIZE - 1, y + BLOCK_SIZE - 1);
setlinestyle(PS_SOLID, 1);
setlinecolor(BLACK);
rectangle(x, y, x + BLOCK_SIZE - 1, y + BLOCK_SIZE - 1);
if (vis[i][j] == 1 && map[i][j] > 0 && map[i][j] < 9) {
settextcolor(BLACK);
setbkmode(TRANSPARENT);
char str[2];
str[0] = map[i][j] + '0';
str[1] = '\0';
outtextxy(x + BLOCK_SIZE / 2 - 4, y + BLOCK_SIZE / 2 - 8, str);
}
if (vis[i][j] == 1 && map[i][j] == 9) {
setfillcolor(RED);
fillcircle(x + BLOCK_SIZE / 2, y + BLOCK_SIZE / 2, BLOCK_SIZE / 3);
}
}
}
}
int game_over()
{
for (int i = 0; i < BOMB_CNT; ++i) {
if (vis[bomb_x[i]][bomb_y[i]] == 1) {
return 1;
}
}
for (int i = 0; i < N; ++i) {
for (int j = 0; j < N; ++j) {
if (vis[i][j] == 0) {
return 0;
}
}
}
return 2;
}
int game_loop()
{
initgraph(N * BLOCK_SIZE, N * BLOCK_SIZE);
init_map();
draw_board();
while (1) {
int x, y;
// 获取鼠标左键点击事件
if (click(&x, &y) == LEFT_BUTTON_DOWN) {
int i = y / BLOCK_SIZE, j = x / BLOCK_SIZE;
if (i >= 0 && i < N && j >= 0 && j < N && vis[i][j] == 0) {
vis[i][j] = 1;
draw_board();
if (game_over() == 1) {
settextcolor(BLACK);
setbkmode(TRANSPARENT);
outtextxy(N * BLOCK_SIZE / 2 - 20, N * BLOCK_SIZE / 2 - 8, "Game Over!");
return 0;
}
if (game_over() == 2) {
settextcolor(BLACK);
setbkmode(TRANSPARENT);
outtextxy(N * BLOCK_SIZE / 2 - 20, N * BLOCK_SIZE / 2 - 8, "You Win!");
return 0;
}
}
}
// 获取鼠标右键点击事件
if (click(&x, &y) == RIGHT_BUTTON_DOWN) {
int i = y / BLOCK_SIZE, j = x / BLOCK_SIZE;
if (i >= 0 && i < N && j >= 0 && j < N && vis[i][j] == 0) {
vis[i][j] = 2;
draw_board();
if (game_over() == 1) {
settextcolor(BLACK);
setbkmode(TRANSPARENT);
outtextxy(N * BLOCK_SIZE / 2 - 20, N * BLOCK_SIZE / 2 - 8, "Game Over!");
return 0;
}
if (game_over() == 2) {
settextcolor(BLACK);
setbkmode(TRANSPARENT);
outtextxy(N * BLOCK_SIZE / 2 - 20, N * BLOCK_SIZE / 2 - 8, "You Win!");
return 0;
}
}
}
delay(10);
}
closegraph();
}
int main()
{
game_loop();
return 0;
}
```
这是一个简单的扫雷游戏,基于C语言编写,使用EasyX图形库绘制游戏界面。在代码中,首先用init_map函数初始化棋盘,随机生成炸弹位置,并计算每个单元格周围的炸弹数。draw_board函数用于绘制游戏界面,包括每个单元格的颜色、数字和图标,以及边框和背景。game_over函数用于判断游戏是否结束,如果有炸弹被点开或者所有非炸弹单元格都被打开,则游戏结束。game_loop函数是游戏主循环,不断获取鼠标事件并更新界面和游戏状态,直到游戏结束。在main函数中调用game_loop函数开始游戏。
阅读全文
相关推荐



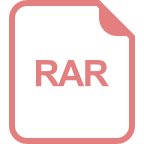
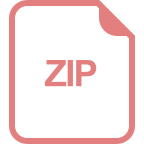
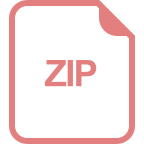
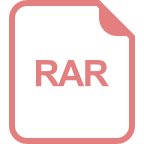
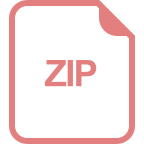
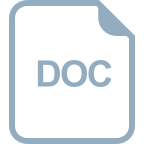
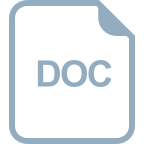
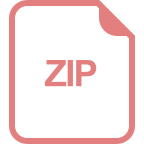
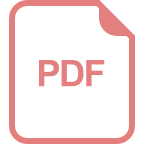
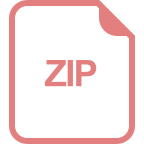