如何使用opencv自动提取图像感兴趣区域并且透视变换
时间: 2023-06-26 08:09:48 浏览: 76
使用OpenCV进行图像感兴趣区域提取和透视变换的步骤如下:
1. 读取图像并转换为灰度图像。
```python
import cv2
import numpy as np
img = cv2.imread('image.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
2. 使用Canny边缘检测算法检测图像边缘。
```python
edges = cv2.Canny(gray, 50, 150, apertureSize=3)
cv2.imshow('Edges', edges)
cv2.waitKey(0)
```
3. 使用霍夫变换检测直线。
```python
lines = cv2.HoughLines(edges, 1, np.pi/180, 200)
for line in lines:
rho, theta = line[0]
a = np.cos(theta)
b = np.sin(theta)
x0 = a*rho
y0 = b*rho
x1 = int(x0 + 1000*(-b))
y1 = int(y0 + 1000*a)
x2 = int(x0 - 1000*(-b))
y2 = int(y0 - 1000*a)
cv2.line(img, (x1, y1), (x2, y2), (0, 0, 255), 2)
cv2.imshow('Lines', img)
cv2.waitKey(0)
```
4. 根据检测到的直线提取感兴趣区域。
```python
# 定义四个顶点的坐标
pts1 = np.float32([[x1, y1], [x2, y2], [x3, y3], [x4, y4]])
# 定义输出图像的大小
pts2 = np.float32([[0, 0], [500, 0], [500, 500], [0, 500]])
# 计算透视变换矩阵
M = cv2.getPerspectiveTransform(pts1, pts2)
# 进行透视变换
dst = cv2.warpPerspective(img, M, (500, 500))
cv2.imshow('Dst', dst)
cv2.waitKey(0)
```
其中,`pts1`是四个顶点的坐标,可以通过手动标注或者其他算法自动获取。`pts2`是输出图像的大小,可以根据需要进行修改。`cv2.getPerspectiveTransform`函数计算透视变换矩阵,`cv2.warpPerspective`函数进行透视变换。
相关推荐
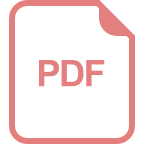
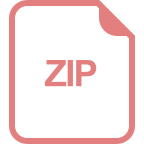
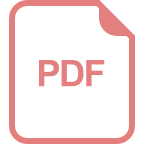
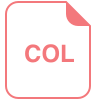
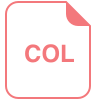
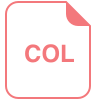
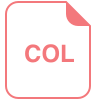
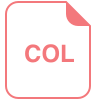








